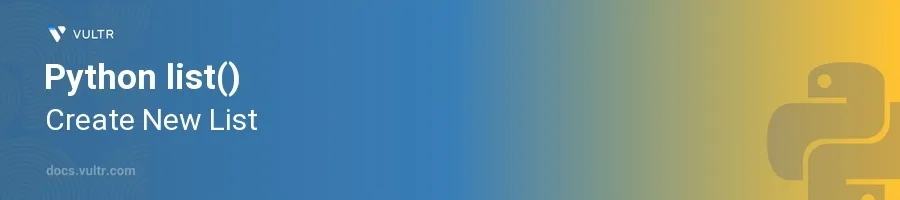
Introduction
The list()
function in Python is a versatile tool used to create new lists from iterables. It's one of the core built-in functions, pivotal for data manipulation and conversion tasks that involve collections in Python programming. Utilizing this function with various data types and structures can significantly enhance data handling efficiency.
In this article, you will learn how to leverage the list()
function to create and convert lists effectively. Explore the foundational techniques to initialize lists from strings, tuples, and other sequences, and see how this function plays a crucial role in data type conversions.
Creating Lists with list()
From Strings
Recognize the usefulness of converting strings to lists to facilitate character manipulation.
Use the
list()
function to convert a simple string into a list of characters.pythonexample_string = "hello" list_from_string = list(example_string) print(list_from_string)
In this code,
list_from_string
will hold the value['h', 'e', 'l', 'l', 'o']
. This transformation allows for easy iteration and manipulation of individual characters.
From Tuples
Understand how tuples, which are immutable, can be made mutable by converting them into lists.
Apply the
list()
function to a tuple to create a modifiable list.pythonexample_tuple = (1, 2, 3, 4) list_from_tuple = list(example_tuple) print(list_from_tuple)
This code snippet changes the tuple
example_tuple
into the listlist_from_tuple
, which is['1', '2', '3', '4']
, allowing for modification of the elements.
From Other Collections
Note that the
list()
function can also convert other iterable types such as sets or dictionaries (keys by default).Convert a set to a list to be able to index the elements.
pythonexample_set = {5, 6, 7, 8} list_from_set = list(example_set) print(list_from_set)
Converting the set to a list
list_from_set
gives you a list[5, 6, 7, 8]
, where indexing and more complex manipulations are now feasible.
From Generators
Consider generator expressions as a memory-efficient way to handle large data iterations.
Use a generator expression with
list()
to convert on-the-fly calculations to a list.pythonsquare_gen = (x**2 for x in range(5)) list_from_gen = list(square_gen) print(list_from_gen)
Here,
list_from_gen
results in[0, 1, 4, 9, 16]
, illustrating how a generator can be used to compute values lazily and then be stored as a list.
Conclusion
The list()
function in Python is a fundamental tool for transforming iterables into lists, enabling a broad range of manipulations and operations. By fully understanding and utilizing this function, you can efficiently handle various data types, implement data transformation pipelines, and improve the flexibility and functionality of your Python code. Whether converting strings for character access or wrapping generators for computed lists, this function provides the necessary capabilities to work effectively with collections in Python.
No comments yet.