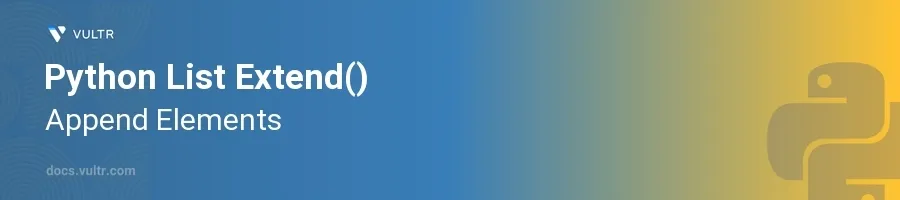
Introduction
The extend()
method in Python is an essential tool for manipulating lists. It allows for the seamless addition of multiple elements from an iterable (like lists, tuples, sets) to the end of a list, thereby extending the list without creating a new one. This functionality is paramount when you need to aggregate data or combine multiple sequences into a single list for further processing.
In this article, you will learn how to effectively use the extend()
method in various practical scenarios. Explore how this method enhances list operations by appending elements from different iterables, comparing its functionality with similar methods, and understand its impact on list memory management.
Using Extend() to Combine Lists
Append Elements from Another List
Start with two lists of elements.
Use the
extend()
method to add all elements from the second list to the first list.pythonlist_one = [1, 2, 3] list_two = [4, 5, 6] list_one.extend(list_two) print(list_one)
This code results in
list_one
being[1, 2, 3, 4, 5, 6]
. The elements oflist_two
are appended tolist_one
.
Extend List with a Tuple
Begin with a list and a tuple.
Apply the
extend()
method to incorporate all tuple elements into the list.pythonnum_list = [1, 2, 3] num_tuple = (4, 5) num_list.extend(num_tuple) print(num_list)
In this scenario,
num_list
updates to[1, 2, 3, 4, 5]
after appending elements fromnum_tuple
.
Extending List with Other Iterables
Using Extend() with a Set
Have a list and a set of unique elements.
Use
extend()
to add elements from the set to the list.pythona_list = [1, 2, 3] a_set = {4, 5, 6} a_list.extend(a_set) print(a_list)
Since sets do not maintain order, the final order of elements in
a_list
post-extension might vary. However,a_list
will contain all elements from both the list and the set.
List Extension with a String
Initialize a list.
Use
extend()
to add each character of a string as an individual element to the list.pythonchar_list = ['a', 'b', 'c'] string = "def" char_list.extend(string) print(char_list)
After execution,
char_list
becomes['a', 'b', 'c', 'd', 'e', 'f']
.
Conclusion
The extend()
method in Python lists is a practical tool to append multiple elements from various iterables to the end of a list. By understanding and utilizing extend()
, manage lists more efficiently and prepare them for complex data processing tasks. This method is ideal for preserving original list IDs (memory location), making it a preferable choice over concatenation in scenarios where list identity matters. Explore using extend()
in diverse programming situations to maintain efficient, clean, and readable code.
No comments yet.