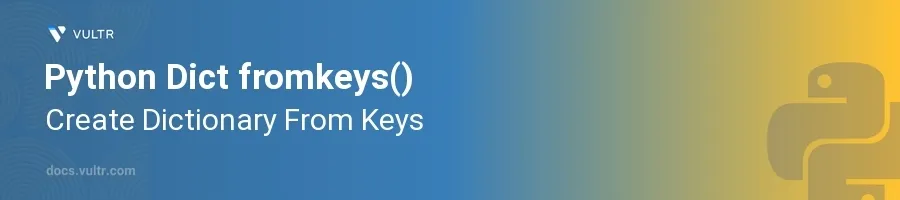
Introduction
The Python dict.fromkeys()
method offers a streamlined way to generate a new dictionary from a list of keys, all initialized to the same value. This method proves incredibly efficient when setting up a dictionary with default values for a predefined set of keys, which is commonly required in data processing and configuration settings tasks.
In this article, you will learn how to leverage the dict.fromkeys()
function to create dictionaries efficiently. You will explore various examples demonstrating its use, including the initialization of keys with default values, customizing values, and handling cases with mutable objects.
Utilizing dict.fromkeys()
Create a Dictionary with Default None Values
Start with a list of keys for which you need a dictionary.
Use
dict.fromkeys()
to create the dictionary where all values areNone
.pythonkeys = ['a', 'b', 'c'] new_dict = dict.fromkeys(keys) print(new_dict)
The output of this code will be
{'a': None, 'b': None, 'c': None}
. This example shows how to use the method to initialize all keys with a default value ofNone
.
Initializing a Dictionary with a Specific Default Value
Define the list of keys and the default value to initialize all keys.
Pass the default value as the second argument to
dict.fromkeys()
.pythonkeys = ['x', 'y', 'z'] default_value = 0 new_dict = dict.fromkeys(keys, default_value) print(new_dict)
When this code executes, it provides the result
{'x': 0, 'y': 0, 'z': 0}
, where each key is initialized to0
.
Handling Mutable Default Values
Recognize the implications of using mutable objects like lists or dictionaries as default values.
To illustrate, try initializing the keys with an empty list.
pythonkeys = [1, 2, 3] new_dict = dict.fromkeys(keys, []) new_dict[1].append(100) print(new_dict)
This will unexpectedly output
{1: [100], 2: [100], 3: [100]}
. All keys share the same list because mutable objects are passed by reference in Python. To avoid this behavior, initialize mutable objects in a distinct manner.
Correct Initialization of Mutable Defaults
Instead of directly passing a mutable object, use a dictionary comprehension to ensure each key gets a separate mutable object.
pythonkeys = [1, 2, 3] new_dict = {key: [] for key in keys} new_dict[1].append(100) print(new_dict)
Here, the result is improved:
{1: [100], 2: [], 3: []}
. Each key now has its own independent list.
Conclusion
The dict.fromkeys()
method in Python is a highly efficient way to create dictionaries from a list of keys, especially when you require all keys to have the same initial value. This method saves time and improves the readability of code that handles dictionary creation with default values. By mastering dict.fromkeys()
and understanding how to properly initialize dictionaries, particularly with mutable objects, you enhance the robustness and reliability of your Python scripts. Use these techniques to ensure that your dictionaries are correctly structured and initialized for various application needs.
No comments yet.