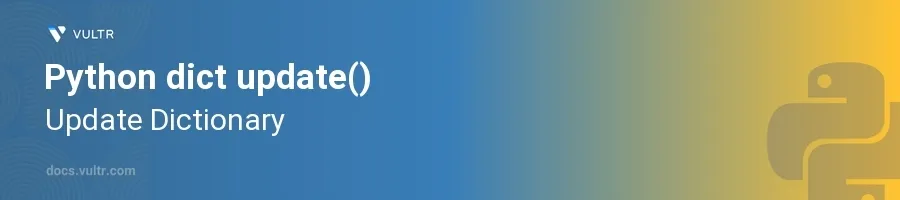
Introduction
The update()
method in Python dictionaries is a powerful tool for merging two or more dictionaries or adding key-value pairs to a dictionary. This method is essential for efficiently managing and manipulating dictionary data in Python, allowing for dynamic and flexible data structures that are crucial in many programming contexts.
In this article, you will learn how to utilize the update()
method to modify dictionaries. Discover how to merge dictionaries, add new entries, and overwrite existing keys with new values, enhancing your capability to handle dictionaries in Python effectively.
Understanding the Update Method
Basic Usage of update()
The update()
method in Python allows you to add key-value pairs to a dictionary or merge dictionaries. It is straightforward to use and modifies the dictionary in-place.
To update a dictionary with another dictionary:
pythondict_a = {'x': 1, 'y': 2} dict_b = {'y': 3, 'z': 4} dict_a.update(dict_b) print(dict_a)
This code updates
dict_a
by adding keys fromdict_b
. Notice that the value of 'y' indict_a
is updated to3
.To update a dictionary with new key-value pairs:
pythondict_a = {'a': 1, 'b': 2} dict_a.update(c=3, d=4) print(dict_a)
Here,
dict_a
is updated with new key-value pairs 'c' and 'd'. This method doesn't require creating a separate dictionary to update existing ones.
Update with Iterable or Iterator
Using an iterable of key-value pairs to update a dictionary:
pythondict_c = {'a': 1, 'b': 2} updates = [('b', 3), ('c', 4)] # list of tuples dict_c.update(updates) print(dict_c)
This updates
dict_c
using a list of tuples where each tuple represents a key-value pair. This approach is useful when you have your updates as a list from another part of your program.
Special Cases
Insert defaults without overwriting existing keys:
pythondict_d = {'e': 5, 'f': 6} defaults = {'f': 7, 'g': 8} for key, value in defaults.items(): dict_d.setdefault(key, value) print(dict_d)
This snippet ensures that while updating
dict_d
withdefaults
, it doesn't overwrite any existing keys.setdefault()
is utilized to only add keys not already present indict_d
.
Conclusion
The update()
method in Python dictionaries simplifies the process of modifying dictionaries by adding or updating entries. This method is versatile, allowing updates from another dictionary, iterable of pairs, or direct key-value arguments. By mastering the update()
method, you ensure more efficient data manipulation and cleaner code. Whether merging data or maintaining flexible and dynamic data structures, update()
enhances your programming toolset in Python.
No comments yet.