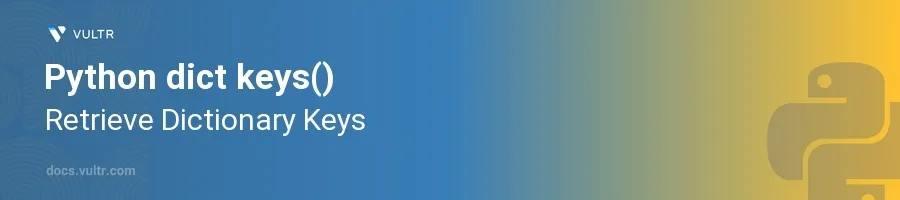
Introduction
The keys()
method in Python is essential for efficiently accessing the keys from a dictionary. This method returns a view object that displays a list of all the keys in the dictionary, which is particularly useful for iterating over keys or checking if specific keys exist in the dictionary.
In this article, you will learn how to use the keys()
method to retrieve keys from a dictionary. You will explore examples that demonstrate basic usage of the method, how to iterate over keys, and how to incorporate these keys into various operations to enhance your data handling capabilities in Python.
Basic Usage of the keys() Method
Retrieve All Keys from a Dictionary
Define a dictionary with some key-value pairs.
Use the
keys()
method to get the keys.pythonmy_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'} keys = my_dict.keys() print(keys)
This code snippet will output the keys of
my_dict
, which are 'name', 'age', and 'city'. Thekeys()
method returns a view of the dictionary's keys, which reflects any changes made to the dictionary.
Convert Keys to a List
Obtain the keys from a dictionary using
keys()
.Use the
list()
function to convert the keys to a list.pythonmy_dict = {'name': 'Bob', 'age': 30, 'city': 'Chicago'} keys_list = list(my_dict.keys()) print(keys_list)
Here, the keys are converted into a list. This is useful for operations that require the keys to be in list form, such as indexing or sorted ordering.
Iterating Over Keys
Iterate Directly Over Keys
Define a dictionary.
Use a
for
loop to iterate over the keys.pythonmy_dict = {'apple': 150, 'banana': 200, 'cherry': 300} for key in my_dict.keys(): print(key, 'has quantity', my_dict[key])
The loop prints each key along with its associated value, demonstrating a common pattern in accessing dictionary items.
Check for a Specific Key
Check if a specific key exists in the dictionary using the
keys()
method.pythonmy_dict = {'red': '#FF0000', 'green': '#00FF00', 'blue': '#0000FF'} if 'green' in my_dict.keys(): print("Green is in the dictionary")
This checks whether 'green' is a key in
my_dict
. Thekeys()
method allows for a direct and easy-to-read syntax for key existence checks.
Conclusion
The keys()
method in Python provides an efficient way to access and manipulate dictionary keys. By understanding how to retrieve, iterate, and convert dictionary keys, you can perform a wide range of tasks more effectively. Whether you're building data structures, performing look-ups, or generating output from dictionary contents, mastering the use of the keys()
method enhances your Python programming skills and leads to cleaner, more efficient code. Through the examples and techniques discussed here, ensure your applications make full use of dictionaries and their capabilities.
No comments yet.