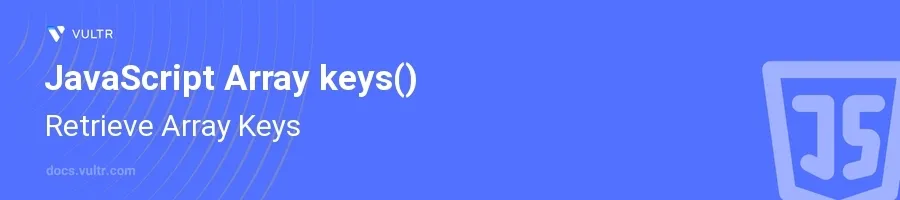
Introduction
The keys()
method in JavaScript is essential for retrieving the index keys from an array. It provides a straightforward way to access these keys as an iterable, allowing you to perform operations like iterations and transformations based exclusively on the indices of elements. This method is particularly useful when you need to loop through indices without directly interacting with the element values.
In this article, you will learn how to use the keys()
method effectively in various scenarios. Explore how to integrate this method into loops, combine it with other array methods, and leverage it to enhance data manipulation and analysis in JavaScript applications.
Retrieving Keys from an Array
Basic Usage of keys()
Create an array.
Call the
keys()
method to get an iterator over the array's keys.Use a
for...of
loop to iterate over the keys.javascriptconst fruits = ['Apple', 'Banana', 'Cherry']; const keys = fruits.keys(); for (const key of keys) { console.log(key); }
This example logs the indices
0
,1
, and2
to the console. Each index corresponds to the position of an item in thefruits
array.
Integrating keys() with Other Array Functions
Utilize the
keys()
method to operate on array indices.Combine
keys()
with themap()
function to manipulate indices.javascriptconst numbers = [10, 20, 30, 40]; const keys = numbers.keys(); const indexesPlusTen = Array.from(keys).map(key => key + 10); console.log(indexesPlusTen);
In this snippet, the array of indices
[0, 1, 2, 3]
is transformed by adding10
to each index, resulting in[10, 11, 12, 13]
.
Using keys() for Conditional Iterations
Employ the
keys()
method for more complex iteration scenarios.Filter array indices based on a condition.
javascriptconst data = [1, null, 3, null, 5]; const keys = data.keys(); const nonNullIndices = Array.from(keys).filter(key => data[key] !== null); console.log(nonNullIndices);
Here,
nonNullIndices
will output[0, 2, 4]
, representing the positions of non-null values in thedata
array.
Conclusion
The keys()
method in JavaScript arrays is a powerful tool for index-based operations. It aids in efficiently handling tasks that require access to array indices without directly manipulating the values. By understanding and implementing the examples provided, you unlock more dynamic ways of data handling and improve the versatility of your JavaScript programming. Harness this method in your projects to optimize array manipulations and enhance code readability and performance.
No comments yet.