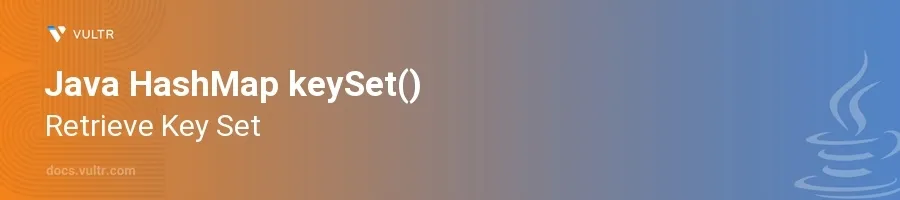
Introduction
The keySet()
method in the Java HashMap
class is a tool for retrieving all the keys from a hashmap. This method returns a set view of the keys contained in the map, which can be iterated over, checked for a particular element, or used to extract values based on these keys. Understanding how to use this function is fundamental when working with hashmaps in Java because it allows developers to access and manipulate sets of keys efficiently.
In this article, you will learn how to effectively utilize the keySet()
method in various programming scenarios. Master the retrieval of all keys from a HashMap, understand how to manipulate these keys, and use the obtained set for further operations such as iterating and removing keys.
Retrieving Key Set from HashMap
Extract Key Set from HashMap
Initialize a
HashMap
.Use the
keySet()
method to retrieve the keys.javaimport java.util.HashMap; import java.util.Set; HashMap<String, Integer> map = new HashMap<>(); map.put("One", 1); map.put("Two", 2); map.put("Three", 3); Set<String> keys = map.keySet(); System.out.println(keys);
This code snippet initializes a
HashMap
with three keys, "One", "Two", and "Three". ThekeySet()
method is then invoked to retrieve the keys from the map which are then printed.
Iterating Over Keys
Retrieve the set of keys from a
HashMap
.Iterate through the set using a for-each loop.
javafor (String key : keys) { System.out.println("Key: " + key + ", Value: " + map.get(key)); }
Here, each key from the set 'keys' is used to fetch the associated value from the map. This demonstrates how to access both keys and values through the key set.
Removing Elements Using Key Set
Understand that modifying the key set directly affects the original map.
Remove an element using an iterator to avoid
ConcurrentModificationException
.javaimport java.util.Iterator; Iterator<String> it = keys.iterator(); while (it.hasNext()) { String key = it.next(); if (key.equals("Two")) { it.remove(); // Removes "Two" from both the set and the map } } System.out.println(map);
By using an iterator, safely remove a key from the set, which simultaneously removes the corresponding entry from the original
HashMap
. This example removes the key "Two".
Conclusion
The keySet()
method from the Java HashMap
class plays a critical role in manipulating and accessing keys. It simplifies tasks such as iterating over keys, performing operations based on keys, and even removing entries effectively while avoiding errors like ConcurrentModificationException
. Adapt the techniques discussed here to enhance your ability to handle key sets in Java applications, ensuring your code is efficient and robust.
No comments yet.