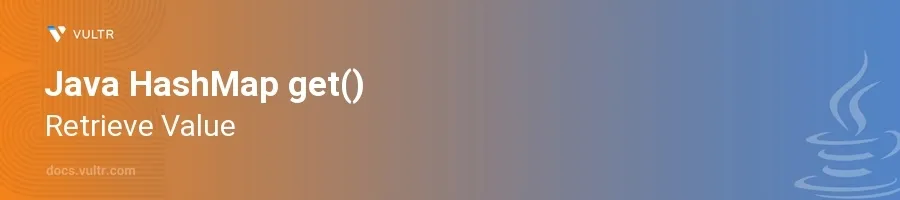
Introduction
The get()
method of a Java HashMap
is a fundamental part of retrieving values associated with a specific key in a map. This method returns the value to which the specified key is mapped, or null
if the map contains no mapping for the key. It's crucial in applications where data needs to be efficiently fetched based on keys, such as in caching implementations, settings configurations, or any system that relies on key-value pairs.
In this article, you will learn how to effectively use the get()
method in a HashMap
. Explore practical examples to understand how to retrieve values, manage the return of null
values, and use this method in real-world Java applications.
Using get() in HashMaps
Basic Value Retrieval
Initialize a
HashMap
and populate it with key-value pairs.Use the
get()
method to retrieve a value based on a key.javaimport java.util.HashMap; HashMap<String, Integer> ageMap = new HashMap<>(); ageMap.put("Alice", 25); ageMap.put("Bob", 30); Integer aliceAge = ageMap.get("Alice"); System.out.println("Alice's age: " + aliceAge);
In this code,
aliceAge
retrieves the age of Alice from theageMap
using theget()
method. Since "Alice" exists as a key, her age,25
, is printed.
Handling Null Values
Understand that
get()
returnsnull
if the key does not exist in the map.Employ a check to see whether the returned value is
null
to handle cases where the key is absent to avoidNullPointerException
.javaInteger missingAge = ageMap.get("Charlie"); if (missingAge != null) { System.out.println("Charlie's age: " + missingAge); } else { System.out.println("Charlie's age is not available."); }
This snippet handles the scenario where the key "Charlie" does not exist in the
ageMap
. It checks if the returned value isnull
before attempting to use it, thus preventing any runtime exceptions.
Using get() with Default Values
Realize the consequence of the default
null
return value and consider usinggetOrDefault()
if Java 8 or later is available.Use
getOrDefault()
to specify a default return value if the key does not exist.javaInteger carolAge = ageMap.getOrDefault("Carol", 28); System.out.println("Carol's age: " + carolAge);
Here, even if "Carol" does not exist in the map, instead of returning
null
, thegetOrDefault()
method returns28
. It provides a more robust and clear approach to handling missing keys.
Conclusion
The get()
method in Java's HashMap
class is essential for efficiently retrieving values linked to specific keys. Mastering this method significantly contributes to enhanced data retrieval operations in Java applications. By following the methods outlined, you optimize data access in your Java applications, handle potential null
values safely, and improve your code's robustness and readability. Embrace these practices to ensure your Java applications are efficient and error-free.
No comments yet.