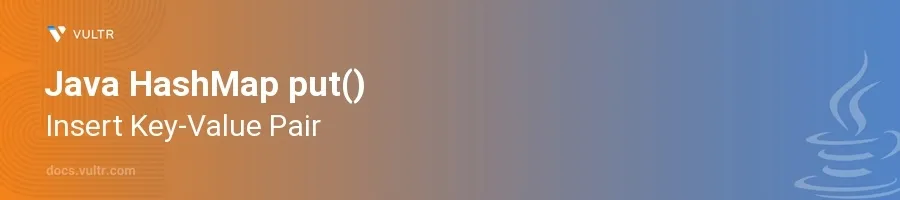
Introduction
The put()
method in Java's HashMap
class plays a crucial role in adding entries to a hash map, allowing you to store key-value pairs efficiently. This method is fundamental for handling associative arrays in Java, where the keys are unique. HashMap
is widely used due to its computational efficiency for insert and retrieve operations, making it an ideal choice for managing datasets where quick lookups are critical.
In this article, you will learn how to leverage the put()
method in various usage scenarios. Understand how this method works under the hood and how to utilize it to manage data dynamically.
Basic Usage of HashMap put()
Insert a Key-Value Pair
Instantiate a
HashMap
.Use the
put()
method to add a key and value.javaimport java.util.HashMap; HashMap<String, Integer> map = new HashMap<>(); map.put("Apple", 10); map.put("Orange", 20);
This code snippet creates a
HashMap
where the keys are types of fruits (as Strings) and the values are their counts (as Integers).
Understanding put() Behavior with Existing Keys
Be aware that adding a key that is already in the map updates the existing key's value.
Demonstrate updating a value in a
HashMap
.javamap.put("Apple", 30);
By calling
put()
with the key"Apple"
again but with a new value,30
, it replaces the old value10
. This functionality is essential for modifying values associated with keys already in the map.
Advanced HashMap Operations Using put()
Handling Null Values and Keys
Recognize that
HashMap
allows null keys and values.Insert entries with null values or keys.
javamap.put(null, 40); map.put("Banana", null);
The
HashMap
now includes an entry with a null key and an entry with a null value. This feature can be useful, but use it judiciously to avoid ambiguity in your data structures.
Return Value of the put() Method
Understand that
put()
returns the previous value associated with the specified key, ornull
if there was no mapping.Capture the return value to check what was replaced.
javaInteger oldValue = map.put("Apple", 25); System.out.println("Previous Value: " + oldValue);
This piece of code outputs
Previous Value: 30
, indicating that30
was the old value associated with"Apple"
before it was updated to25
.
Conclusion
The put()
method of the HashMap
class in Java provides a powerful way to manage key-value pairs. Its ability to adjust an existing key's value or add new key-value pairs makes it invaluable for data management and manipulation in Java applications. By fully understanding and utilizing the put()
method, effectively manage your data in Java applications to ensure efficiency and clarity in your code. Use the examples provided as a guide for implementing HashMap
in your projects, and take advantage of its robust functionality to streamline your data handling tasks.
No comments yet.