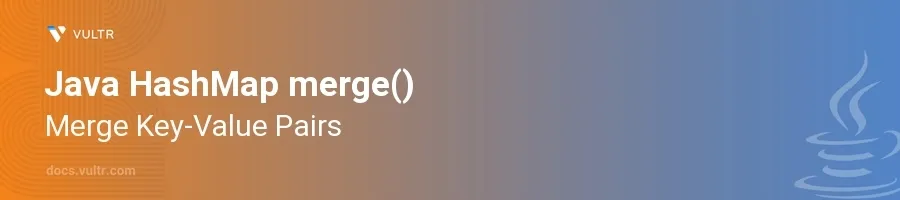
Introduction
The merge()
method in Java's HashMap
is a powerful utility for combining key-value pairs effectively. This method simplifies the process of updating HashMap
values based on specified rules, especially useful in scenarios where you need to cumulatively combine or modify values associated with identical keys. For example, it can be used in data aggregation tasks, counters, or when merging settings or configurations.
In this article, you will learn how to effectively utilize the merge()
method in various scenarios involving HashMaps
. Discover how this function can streamline code when working on data aggregation and when you need to handle conflicts between values efficiently.
Understanding HashMap merge()
Basic Usage of merge()
Understand the function signature of
merge()
.Use
merge()
to add or update entries in aHashMap
.javaHashMap<String, Integer> map = new HashMap<>(); map.put("a", 5); map.merge("a", 3, Integer::sum); System.out.println(map.get("a")); // Outputs 8
This code demonstrates the basic use of
merge()
. If the key "a" is found, it adds the given value (3) to the existing value (5), usingInteger::sum
as the merging function.
Handling Absent Keys Using merge()
Realize that
merge()
can also handle keys not currently present in theHashMap
.Employ
merge()
to effortlessly add new key-value pairs.javaHashMap<String, Integer> map = new HashMap<>(); map.merge("b", 1, Integer::sum); System.out.println(map.get("b")); // Outputs 1
Here, since "b" is not yet in the map,
merge()
inserts the new key "b" with the provided value 1.
Using Custom Merge Functions
Explore using custom merge functions beyond the common arithmetic operations.
Apply
merge()
with a custom lambda expression to handle specific merging logic.javaHashMap<String, Integer> map = new HashMap<>(); map.put("c", 2); map.merge("c", 3, (oldVal, newVal) -> oldVal * newVal); System.out.println(map.get("c")); // Outputs 6
In this snippet, the merging function multiplies the existing value with the new value, thus updating "c" from 2 to 6 (2 * 3).
Advanced Use-Cases of HashMap merge()
Merging Maps with Stream API
Exploit Java Streams to merge one map into another.
Utilize
forEach()
in conjunction withmerge()
for efficient map merging.javaHashMap<String, Integer> map1 = new HashMap<>(); HashMap<String, Integer> map2 = new HashMap<>(); map1.put("a", 5); map2.put("a", 3); map2.put("b", 7); map2.forEach((key, value) -> map1.merge(key, value, Integer::sum)); System.out.println(map1); // Outputs {a=8, b=7}
Using
forEach()
frommap2
, each key-value pair is merged intomap1
using the sum function for values with matching keys.
Conclusion
The merge()
method in Java's HashMap
enhances data manipulation capabilities by providing a structured way to handle the merging of key-value pairs based on custom rules. Whether adding new entries or updating existing ones with complex logic, merge()
simplifies operations and ensures efficient and readable code. Adapt the examples provided to match specific requirements, from simple counting tasks to sophisticated data merging scenarios, streamlining your Java data handling processes.
No comments yet.