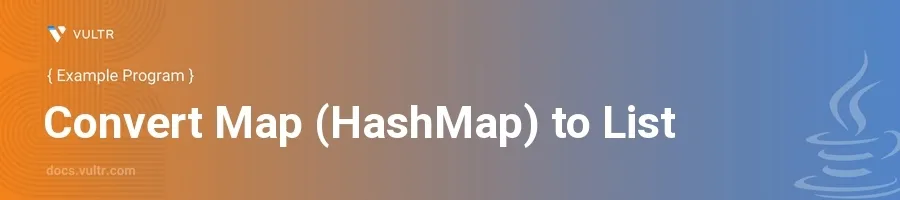
Introduction
In Java, maps and lists are two of the most commonly used data structures in the collections framework. Maps, such as HashMap
, store items in key-value pairs, whereas lists store items in an ordered sequence. There are scenarios in software development where you might need to convert a Map
to a List
. For example, you might want to sort the keys or values of a map, or perhaps you need a list representation for APIs that require a list input.
In this article, you will learn how to convert a Map
(specifically HashMap
) into a List
. Gain insights into extracting either keys, values, or both from a map and storing them into a List
. Demonstrate the conversion process through practical coding examples that you can readily implement in your projects.
Extracting Keys from a Map
Convert Map Keys to List
Create a
HashMap
and populate it with sample data.Utilize the
keySet()
method to extract the keys, then construct aList
using theArrayList
constructor.javaimport java.util.*; public class Main { public static void main(String[] args) { Map<String, Integer> map = new HashMap<>(); map.put("one", 1); map.put("two", 2); map.put("three", 3); List<String> keysList = new ArrayList<>(map.keySet()); System.out.println(keysList); } }
The code above creates a
HashMap
and retrieves the keys using thekeySet()
method. These keys are then stored in anArrayList
, which is subsequently printed.
Extracting Values from a Map
Convert Map Values to List
Initialize a
HashMap
and include some key-value pairs.Use the
values()
method to get the values from the map and direct these into a newArrayList
.javaimport java.util.*; public class Main { public static void main(String[] args) { Map<String, Integer> map = new HashMap<>(); map.put("a", 100); map.put("b", 200); map.put("c", 300); List<Integer> valuesList = new ArrayList<>(map.values()); System.out.println(valuesList); } }
In this snippet,
values()
is called on theHashMap
to obtain a collection of values, which are then stored in anArrayList
.
Extracting Both Keys and Values
Convert Both Keys and Values to List of Entries
Start with a populated
HashMap
.Use the
entrySet()
method to get the set of entries.Stream the entries to convert each entry to a custom object or a string representation.
javaimport java.util.*; import java.util.stream.Collectors; public class Main { public static void main(String[] args) { Map<String, Integer> map = new HashMap<>(); map.put("x", 24); map.put("y", 25); map.put("z", 26); List<String> entriesList = map.entrySet() .stream() .map(entry -> entry.getKey() + "=" + entry.getValue()) .collect(Collectors.toList()); System.out.println(entriesList); } }
This example uses Java Streams to transform each map entry into a string combining key and value, and then collects these strings into a list.
Conclusion
Converting a HashMap
to a List
in Java is a straightforward process thanks to the collections framework and Java Stream API. Depending on whether you need keys, values, or both in list form, Java provides efficient methods such as keySet()
, values()
, and entrySet()
to aid in these conversions. By following the demonstrated examples, you enhance your ability to manipulate and adapt data structures in Java, ensuring your code is versatile and capable of meeting various functional requirements. Whether for data manipulation, API interaction, or any other reason, mastering these conversions prepares you to handle diverse programming challenges effectively.
No comments yet.