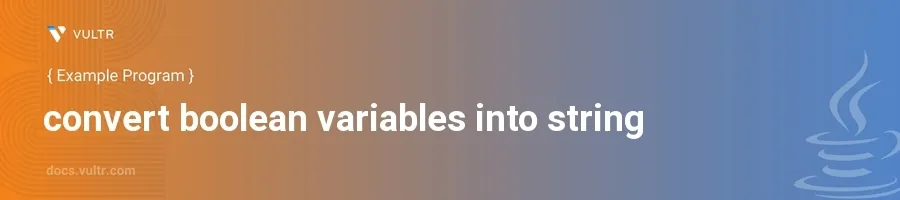
Introduction
Converting data types is a common task in programming, particularly when it comes to manipulating and displaying variables in different formats. In Java, converting boolean values to Strings is a straightforward process, but it's essential to understand various ways to achieve this to best suit your application's requirements.
In this article, you will learn how to convert boolean variables into strings using Java. Explore several methods, including the use of standard library functions and manual conversion techniques, to efficiently transform and manipulate boolean data.
Basic Conversion Using toString()
Convert Directly Using Boolean.toString()
Start with a boolean variable.
Use the
Boolean.toString()
method to convert the boolean to a string.javaboolean myBool = true; String boolAsString = Boolean.toString(myBool); System.out.println(boolAsString);
This method directly converts the
myBool
boolean variable to a string usingBoolean.toString()
method and displaystrue
orfalse
as a string.
Advantages of Using Boolean.toString()
- Simplicity and directness in conversion.
- Automatic handling of
true
andfalse
values.
Using String.valueOf() Method
Conversion with String.valueOf()
Declare a boolean variable.
Convert the boolean to a string using
String.valueOf()
.javaboolean anotherBool = false; String boolString = String.valueOf(anotherBool); System.out.println(boolString);
The
String.valueOf()
method is used here to convert the booleananotherBool
into the stringboolString
. This will output 'false' as a string.
Benefits of String.valueOf()
- Consistency in style when used with other data types.
- Avoids null exceptions as every input is handled gracefully.
Manual Conversion Using Ternary Operator
Implementing Ternary Operator for Conversion
Initialize a boolean variable.
Use a ternary operator to decide the output string based on the boolean's value.
javaboolean condition = true; String conditionAsString = condition ? "true" : "false"; System.out.println(conditionAsString);
In this snippet, the ternary operator checks the
condition
boolean. Ifcondition
is true, it assigns"true"
toconditionAsString
; otherwise, it assigns"false"
.
Why Use a Ternary Operator?
- Provides more control over the output format.
- Allows inline conversion and assignment.
Conclusion
Converting boolean values to strings in Java can be done effectively using several methods including Boolean.toString()
, String.valueOf()
, and the ternary operator. Each method has its use cases depending on the required code clarity and complexity. While Boolean.toString()
and String.valueOf()
offer straightforward conversions, using a ternary operator gives you more control over the output, allowing for custom string results based on the boolean's value. Master these techniques to enhance the data handling capabilities of your Java applications.
No comments yet.