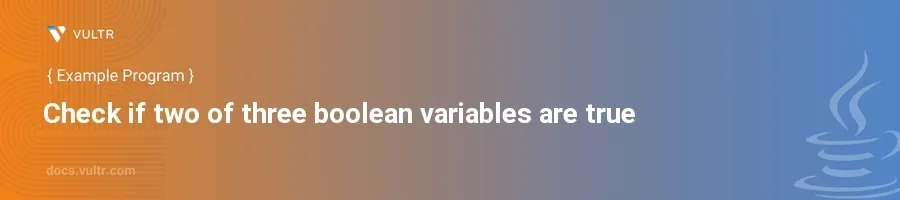
Introduction
In Java, managing the logical conditions involving boolean variables is a common task, especially when you need to evaluate multiple conditions to determine the flow of execution in programs. This type of logical decision making can be crucial for everything from simple utility programs to complex business logic.
In this article, you will learn how to check if two out of three boolean variables are true in a Java program. Explore different methods and examples that illustrate how to effectively handle such conditions using basic logical operators and conditional statements.
Implementing with Logical Operators
Using AND and OR Operators
To determine if exactly two of the three boolean variables are true, you can combine the AND (&&
) and OR (||
) operators in a way that checks each pair of variables while ensuring that not all three variables are true. This technique is both simple and effective for most use cases.
Define three boolean variables.
Use a logical expression that checks for any two variables being true but not all three.
javaboolean var1 = true; boolean var2 = false; boolean var3 = true; boolean twoTrue = (var1 && var2 && !var3) || (var1 && !var2 && var3) || (!var1 && var2 && var3); System.out.println(twoTrue);
In the provided code, each pair
(var1, var2)
,(var1, var3)
, and(var2, var3)
is evaluated in conjunction with the negation of the third variable. This approach ensures that exactly two variables are true.
Evaluating with XOR Exclusive OR Operator
The Exclusive OR (XOR) operator (^
) can also be used to determine if exactly two variables are true by checking that only one variable among the three is false.
Initialize the boolean variables.
Write a condition using XOR that ensures only one of the variables is different (i.e., false).
javaboolean var1 = true; boolean var2 = true; boolean var3 = false; boolean twoTrue = (var1 ^ var2 ^ var3) && (var1 || var2 || var3); System.out.println(twoTrue);
This code checks that exactly one variable is false. The added condition
(var1 || var2 || var3)
ensures that not all variables are false, thus confirming at least two are true.
Counting True Values Method
Using a Count Variable
For situations where scalability is a concern (more than three variables), counting the number of true
values in a more dynamic way can be advantageous. Here, use a simple counter to tally the number of true variables.
Declare and initialize the boolean variables.
Create an integer counter.
Increment the counter for each true variable.
Check if the counter equals 2.
javaboolean var1 = false; boolean var2 = true; boolean var3 = true; int trueCount = 0; if (var1) trueCount++; if (var2) trueCount++; if (var3) trueCount++; boolean twoTrue = (trueCount == 2); System.out.println(twoTrue);
The code snippet precisely counts the number of
true
values. The final check confirms whether exactly two variables are true.
Conclusion
Checking if two out of three boolean variables are true in Java requires understanding and applying logical operators effectively. The methods discussed range from direct logical expressions using AND, OR, and XOR operators to counting the number of true values in more dynamic or scalable scenarios. Choose the approach that best fits the complexity and needs of your specific application to maintain clarity and efficiency in your code. With the outlined examples and explanations, manage multiple boolean conditions with confidence and precision in your Java programs.
No comments yet.