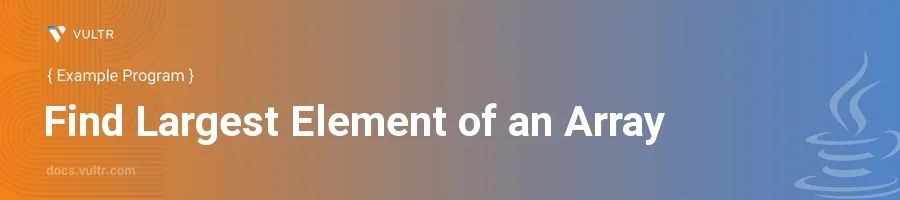
Introduction
In Java, finding the largest element or number in an array is a common task that helps in understanding basic array operations and control structures such as loops and conditional statements. This operation is fundamental in various algorithmic challenges and can serve as a stepping stone to more complex data manipulation techniques.
In this article, you will learn how to identify the largest element within an array using different methods. Explore how to implement this in a straightforward manner and through more enhanced techniques that optimize performance and readability by understanding how to find maximum element in an array in java.
Finding the Largest Element or Number in Array Using Loop
Basic Iteration Method
Initialize an array with some values.
Set a variable to store the maximum value, typically starting with the first element of the array.
Iterate through the array, comparing each element with the current maximum and update the maximum if a larger element is found.
javapublic class Main { public static void main(String[] args) { int[] numbers = {5, 34, 78, 2, 45, 1, 99, 23}; int max = numbers[0]; // Start with the first element for (int i = 1; i < numbers.length; i++) { if (numbers[i] > max) { max = numbers[i]; } } System.out.println("Largest element is: " + max); } }
This code iterates through the
numbers
array and continuously updates themax
variable whenever a larger element is found. By the end of the loop,max
holds the largest value, which is a common approach used to find largest number in array Java using for loop.
Stream API Method for Conciseness
Using Streams to Find Maximum
Import the Stream API.
Convert the array to a stream.
Apply the
max()
method provided by the Stream API, which is more concise and readable for those familiar with functional programming.javaimport java.util.Arrays; public class Main { public static void main(String[] args) { int[] numbers = {5, 34, 78, 2, 45, 1, 99, 23}; int max = Arrays.stream(numbers).max().getAsInt(); // Directly find max System.out.println("Largest element is: " + max); } }
After converting the array to a stream with
Arrays.stream(numbers)
, themax()
method computes the maximum element. It returns anOptionalInt
, sogetAsInt()
is used to retrieve the value as an integer—this is a typical method used to find max element in array java.
Conclusion
Finding the largest element in an array in Java can be achieved using traditional loops or more modern approaches like the Stream API. Utilize loops for educational purposes and direct control over the process, or adopt Stream API for more succinct and readable code. By mastering these methods, such as how to find largest number in array java, enhance array manipulation capabilities and improve the efficiency and readability of Java programs.
No comments yet.