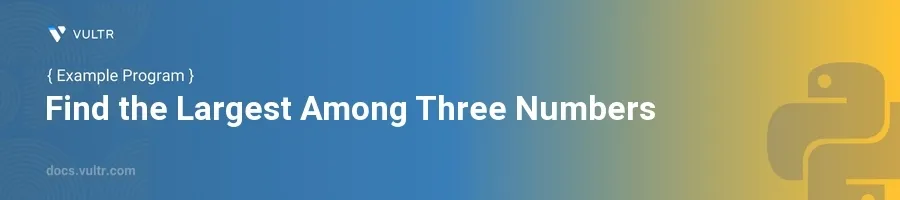
Introduction
Determining the largest number among three candidates is a common problem that can be solved efficiently using Python. This task often appears in introductory programming courses and exercises, highlighting key programming concepts such as conditional statements and comparisons.
In this article, you will learn how to write a program to find the largest of three numbers in python. From using basic if-else structures to implementing more Pythonic solutions, this guide provides clear examples and explanations to ensure you can apply these methods in your projects or learning exercises.
Find the Largest of Three Numbers in Python Using Traditional if-else
Steps to Implement the Solution
Set up three variables to hold the numbers you want to compare.
Use nested if-else statements to determine the largest number.
python# Variables holding the numbers num1 = 5 num2 = 14 num3 = 9 # Comparing the numbers using if-else if num1 >= num2 and num1 >= num3: largest = num1 elif num2 >= num1 and num2 >= num3: largest = num2 else: largest = num3 print("The largest number is:", largest)
This script initializes three variables and uses a series of if-else conditions to determine which number is the largest. The result is printed directly.
Largest of Three Numbers in Python Using the max() Function
Simplifying with Built-In Functionality
Initialize the numbers.
Use the
max()
function to find the largest number in a single line.python# Variables holding the numbers num1 = 5 num2 = 14 num3 = 9 # Finding the largest number using max() largest = max(num1, num2, num3) print("The largest number is:", largest)
The
max()
function simplifies finding the largest number by handling the comparisons internally. This approach is not only shorter but also more readable and less error-prone.
Greatest of Three Numbers in Python Using a Custom Function
Creating a Reusable Solution
Define a function that accepts three numbers as parameters.
Use the
max()
function within this custom function for a clean solution.pythondef find_largest(n1, n2, n3): return max(n1, n2, n3) # Example usage of the function largest = find_largest(5, 14, 9) print("The largest number is:", largest)
By implementing a custom function, you encapsulate the logic for finding the largest number, making the code reusable and modular. This is useful in larger programs where you might need to perform this operation multiple times.
Conclusion
Choosing the right approach to find the largest among three numbers in Python involves understanding both basic programming constructs like if-else and utilizing Python's built-in functions for concise solutions. Whether you opt for detailed manual comparisons or streamlined built-in functions, Python provides the flexibility to approach the problem in various ways. Adopting these techniques will enhance your problem-solving skills in Python, ensuring robust and efficient code in your projects.
No comments yet.