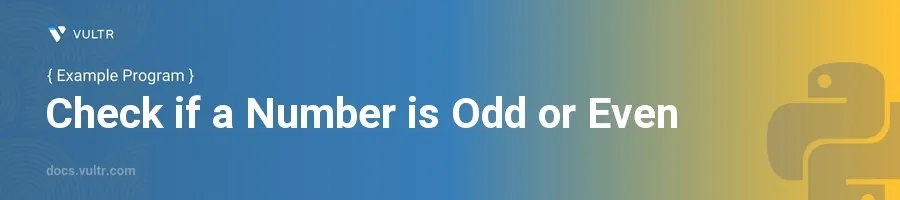
Introduction
Determining whether a number is odd or even is a foundational concept in programming and mathematics, and it is especially straightforward to implement in Python. This binary check serves as an excellent example to illustrate simple conditional statements and is often one of the first examples taught to new programmers.
In this article, you will learn how to use Python to create a program that checks if a given number is odd or even. You will walk through setting up the function to perform this task and explore several examples that test different numbers. By the end of this article, gain the skills to implement similar checks in Python applications efficiently.
Creating the Function to Check Odd and Even Numbers
Define the Function
Start by defining a function called
check_odd_even
.Inside the function, use the modulus operator (
%
) to determine the remainder when the number is divided by 2.If the remainder is 0, the number is even; otherwise, it is odd.
pythondef check_odd_even(num): if num % 2 == 0: return "Even" else: return "Odd"
This function receives a number as an argument and returns "Even" if the number is divisible by 2 with no remainder, indicating it is even. Otherwise, it returns "Odd".
Utilize the Function with Multiple Examples
To illustrate the use of the
check_odd_even
function, input a series of different numbers.pythonprint(check_odd_even(2)) # Should output 'Even' print(check_odd_even(3)) # Should output 'Odd' print(check_odd_even(10)) # Should output 'Even' print(check_odd_even(21)) # Should output 'Odd'
In this section, the
check_odd_even
function is called multiple times with different numbers. Each call evaluates whether the input number is odd or even based on the logic implemented in the function.
Handling Edge Cases
Zero and Negative Numbers
Test the function with zero and negative numbers to ensure it handles all possible integer inputs correctly.
Implement the test cases using the same function.
pythonprint(check_odd_even(0)) # Should output 'Even' print(check_odd_even(-1)) # Should output 'Odd' print(check_odd_even(-2)) # Should output 'Even'
Zero is considered even because zero divided by any positive integer results in a remainder of zero. Negative numbers can also be either odd or even, depending on their divisibility by 2. In these test cases, the function correctly identifies zero as even and correctly assesses the parity of negative integers.
Conclusion
Building a Python program to check if a number is odd or even highlights how simple operations, like modulus, conditionally branch code execution. By understanding and implementing these foundational concepts, you enhance the capability to solve more complex problems. Now, feel confident applying similar checks and basic function creation in different Python applications, ensuring versatility in your programming skills.
No comments yet.