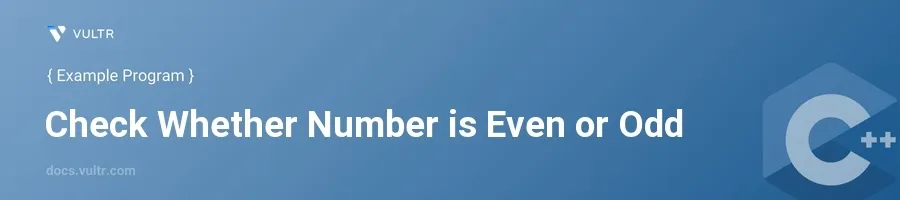
Introduction
Determining whether a number is even or odd is a fundamental concept in programming, often explored when first learning a language like C++. This simple classification task involves checking the remainder of a number when divided by 2. If the remainder is zero, the number is even; otherwise, it's odd. This basic concept helps in understanding conditional statements and arithmetic operations in C++.
In this article, you will learn how to create a C++ program to check if a number is even or odd. Explore the implementation of this program through various examples, which include using conditional (if-else) statements and the ternary operator for different scenarios.
Implementing Basic Check with if-else
Before diving into specific examples, understand the foundational approach to determine the evenness or oddness of a number using conditional statements.
Basic Even/Odd Check
Prompt the user to input a number.
Use an if-else statement to determine if the number is even or odd.
cpp#include<iostream> using namespace std; int main() { int num; cout << "Enter an integer: "; cin >> num; if (num % 2 == 0) { cout << num << " is even." << endl; } else { cout << num << " is odd." << endl; } return 0; }
In this program:
- The modulus operator
%
checks the remainder whennum
is divided by 2. - According to the condition
num % 2 == 0
, if the remainder is zero, it prints that the number is even; otherwise, it prints that the number is odd.
- The modulus operator
Utilizing the Ternary Operator
For a more concise implementation, the ternary operator can be used. This operator allows embedding the if-else logic directly into the output statement.
Compact Even/Odd Check Using Ternary Operator
Recognize that the ternary operator
?:
can simplify the code.Implement the even/odd check in a single line within the output command.
cpp#include<iostream> using namespace std; int main() { int num; cout << "Enter an integer: "; cin >> num; string result = (num % 2 == 0) ? "even" : "odd"; cout << num << " is " << result << "." << endl; return 0; }
Here:
- The expression
(num % 2 == 0) ? "even" : "odd"
directly computes whethernum
is even or odd. It assigns "even" or "odd" to the variableresult
based on the modulus operation. - This approach reduces the lines of code and enhances readability when conditions are simple.
- The expression
Handling Multiple Numbers
Sometimes, the need arises to check the evenness or oddness of multiple numbers, such as in a list or array.
Multi-Number Check in Loops
Incorporate loops to handle the even/odd check across multiple numbers.
Array and loop usage can facilitate this process.
cpp#include<iostream> using namespace std; int main() { int numbers[] = {10, 23, 46, 57, 89}; int size = sizeof(numbers) / sizeof(numbers[0]); for(int i = 0; i < size; i++) { cout << numbers[i] << " is " << ((numbers[i] % 2 == 0) ? "even" : "odd") << "." << endl; } return 0; }
In this iteration:
- An array
numbers
of integer type is specified with five numbers. - A for-loop cycles through each element of the array and prints whether it is even or odd using the same ternary operator used previously.
- An array
Conclusion
Creating a C++ program to check if a number is even or odd is a basic yet crucial exercise to grasp handling conditional statements and arithmetic operations. Whether using traditional if-else structures or employing more succinct methods like the ternary operator, you equip yourself with versatile approaches to simple decision-making tasks in coding. Adapt these methods to enhance both functionality and readability in your programs, ensuring robust foundations for further C++ programming challenges.
No comments yet.