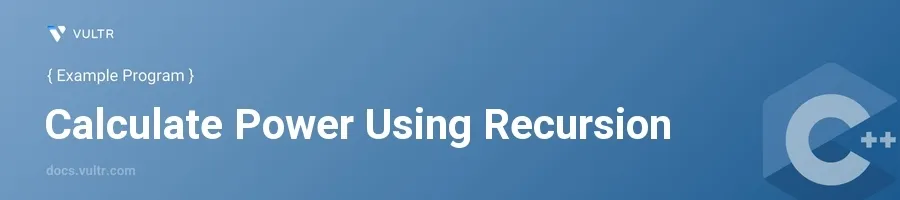
Introduction
Recursion in C++ offers a powerful technique for solving problems that can be broken down into smaller sub-problems of the same type. One classic application of recursion is in calculating exponentiation, where the power of a number is computed. The principle behind recursion is simple: the problem is divided into a base case that can be solved directly and a recursive case that calls the same operation.
In this article, you will learn how to implement a function in C++ that calculates the power of a number using recursion. Explore various examples that demonstrate how recursion can simplify problems while maintaining clarity and efficiency in your code. Special attention is given to understanding the recursive call and how it results in the final computed value. Implementing a recursive power function in C++ is a fundamental exercise that enhances your grasp of recursive algorithms and their applications.
Implementing Power Calculation Using Recursion
Recursion allows problems to be solved by breaking them down into simpler instances of the same problem. Calculating the power of a number, ( a^n ) (where ( a ) is the base and ( n ) is the exponent), can efficiently be managed through recursion.
Basic Recursive Function for Power Calculation
Start by defining a function that will recursively calculate the power of a number.
cppint power(int base, int exponent) { if (exponent == 0) return 1; // Base case: any number to the power of 0 is 1 else return base * power(base, exponent - 1); // Recursive case }
- In the code above, the base case for the recursion is when
exponent
is 0. Here, the function simply returns 1 because any number to the power of zero is 1. - In a power function using recursion, the recursive case reduces the problem size by decrementing the exponent by one and then multiplying the base with the result of the recursive call.
- In the code above, the base case for the recursion is when
Optimizing the Function with Even Exponents
Modify the function so it handles cases where the exponent is even more efficiently by using the property ( a^n = (a^{n/2})^2 ).
cppint powerOptimized(int base, int exponent) { if (exponent == 0) return 1; int halfPower = powerOptimized(base, exponent / 2); if (exponent % 2 == 0) return halfPower * halfPower; // Efficient calculation for even exponent else return base * halfPower * halfPower; // For odd exponents }
- By calculating the power of the base raised to half the exponent and then squaring it, the number of multiplicative operations is reduced, particularly when dealing with large exponents.
- This optimization significantly reduces the time complexity from ( O(n) ) to ( O(\log n) ) where ( n ) is the exponent.
Edge Cases and Negative Exponents
Improve the function to handle negative exponents.
cppdouble powerWithNegatives(int base, int exponent) { if (exponent == 0) return 1; // Base case else if (exponent < 0) return 1 / powerWithNegatives(base, -exponent); // Handling negative exponents else { double halfPower = powerWithNegatives(base, exponent / 2); if (exponent % 2 == 0) return halfPower * halfPower; else return base * halfPower * halfPower; } }
- The function now correctly calculates the power for negative exponents by taking the reciprocal of the power calculated with the positive equivalent of the exponent. This efficiently extends the utility of the power function.
Conclusion
Recursion is a distinctly powerful concept in programming that allows for solving problems in a clear and effective manner. In this article, you explored implementing a recursive function to compute the power of a number using C++. By breaking down the problem and employing recursion, the code maintains readability and efficiently manages computation even with optimizations for special cases like even and negative exponents. These examples not only demonstrate recursion but also optimize the computation, making it suitable for larger inputs. Utilizing these concepts in various applications ensures your code is both powerful and efficient.
No comments yet.