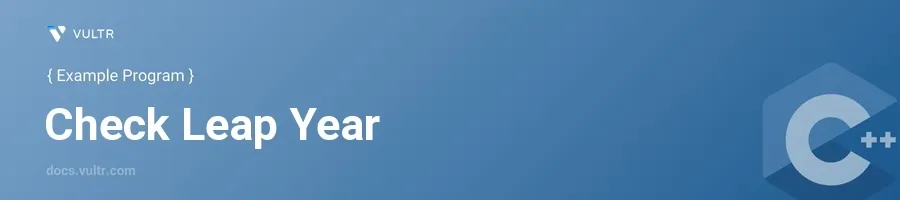
Introduction
Determining whether a year is a leap year is a common task in software development, particularly when designing calendars and scheduling applications. Leap years, which include an additional day, must be correctly identified to ensure accurate date-related calculations. This involves not only understanding the rules that define a leap year but also how to effectively implement these rules in programming, with C++ being an ideal choice due to its performance and control over system resources.
In this article, you will learn how to implement a C++ program that can determine whether a given year is a leap year. You'll explore detailed examples that demonstrate the process, ensuring that you can apply these concepts immediately to your own C++ projects.
Understanding Leap Year Rules
Before delving into coding, it's crucial to grasp the criteria that define a leap year:
- A year that is divisible by 4 is a leap year.
- However, if the year is also divisible by 100, it is not a leap year, unless:
- The year is also divisible by 400, in which case it is a leap year.
These rules ensure that the year aligns more accurately with the astronomical year.
C++ Program Examples
Example 1: Basic Leap Year Checker
This example demonstrates a simple function to check if a year is a leap year.
Define a function
isLeapYear
that takes an integer year as a parameter.Implement the leap year rules within this function using conditional statements.
cpp#include <iostream> using namespace std; bool isLeapYear(int year) { if (year % 400 == 0) return true; if (year % 100 == 0) return false; if (year % 4 == 0) return true; return false; } int main() { int year; cout << "Enter a year: "; cin >> year; bool leap = isLeapYear(year); if (leap) cout << year << " is a leap year." << endl; else cout << year << " is not a leap year." << endl; return 0; }
This C++ code starts by defining the
isLeapYear
function that applies the leap year conditions using an efficient order of checks: first for divisibility by 400, then 100, and finally 4. Themain
function then uses this logic to check a user-inputted year and displays the result.
Example 2: Using Function to Handle Range of Years
Expanding on the previous example, here's how you can check for leap years over a range of years.
Utilize the
isLeapYear
function created in Example 1.Create a loop in the
main
function to check each year in a specified range.cpp#include <iostream> using namespace std; bool isLeapYear(int year) { if (year % 400 == 0) return true; if (year % 100 == 0) return false; if (year % 4 == 0) return true; return false; } int main() { int startYear, endYear; cout << "Enter the start year: "; cin >> startYear; cout << "Enter the end year: "; cin >> endYear; for (int year = startYear; year <= endYear; year++) { if (isLeapYear(year)) cout << year << " is a leap year." << endl; else cout << year << " is not a leap year." << endl; } return 0; }
This example allows the user to input a range, starting with
startYear
and ending withendYear
. The program then iterates through each year in the range, checks if it's a leap year using theisLeapYear
function, and outputs the result.
Conclusion
Writing a C++ program to check for leap years helps reinforce your understanding of conditional logic and function use in programming. By implementing the given examples, you can efficiently determine whether a year qualifies as a leap year based on the established rules. This capability is essential in various real-world applications, from handling date and time data accurately to ensuring the correctness of calendrical computations in software development. Keep experimenting with different implementations and scenarios to strengthen your grasp of C++ programming principles.
No comments yet.