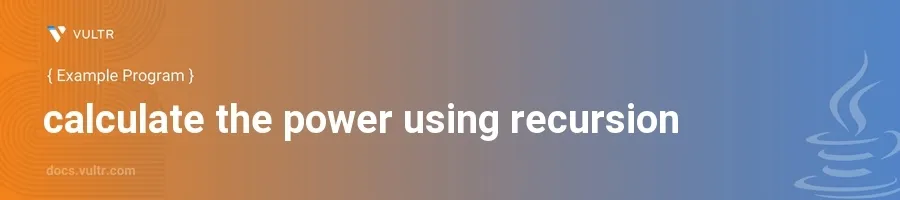
Introduction
In Java, recursion is a process where a method calls itself continuously. A typical application of recursion in Java is calculating the power of a number, where the power function multiplies the base by itself a number of times equal to the exponent. This process can be efficiently implemented using recursive techniques, making the code both interesting and efficient to execute.
In this article, you will learn how to implement a power calculation using recursion in Java. You will explore various examples that demonstrate how to calculate different powers of integers, including edge cases like zero and negative powers, thereby covering a comprehensive set of scenarios.
Implementing Power Calculation Using Recursion
Basic Power Function
Define a recursive method
power
that takes the base and the exponent as arguments.Implement the base case where if the exponent is zero, return 1 since any number raised to the power of zero is 1.
Define the recursive case that calls the
power
method within itself to multiply the base for the number of times defined by the exponent.javapublic class PowerCalculator { public static double power(double base, int exponent) { if (exponent == 0) { return 1; } return base * power(base, exponent - 1); } }
In this code,
power
checks if theexponent
is zero; if not, it recursively calls itself while decrementing theexponent
. Each time, it multiplies thebase
until theexponent
becomes zero, at which point 1 is returned, and the multiplications are resolved backward.
Handling Negative Exponents
Adapt the
power
method to handle negative exponents by taking the reciprocal of the base for each recursive call.Modify the method to return
1 / power(base, -exponent)
when the exponent is negative.javapublic static double power(double base, int exponent) { if (exponent == 0) { return 1; } else if (exponent < 0) { return 1 / power(base, -exponent); } return base * power(base, exponent - 1); }
With this implementation, when the
exponent
is negative, the method computespower(base, -exponent)
, taking the reciprocal of the result. This approach effectively calculates the power for negative exponents.
Main Method to Test Power Function
Add a
main
method to thePowerCalculator
class.Use the
power
method to compute various powers of numbers and print the results to verify the correct functionality.javapublic static void main(String[] args) { System.out.println("2 raised to the power of 3 is " + power(2, 3)); System.out.println("5 raised to the power of 0 is " + power(5, 0)); System.out.println("3 raised to the power of -4 is " + power(3, -4)); }
This
main
method tests thepower
function with different bases and exponents, printing out results that include positive, zero, and negative powers.
Conclusion
Recursion in Java offers a robust and elegant method for solving problems such as calculating the power of a number. By understanding and implementing recursive methods, you can handle complex calculations in a relatively simple way. The above examples provide a foundation for using recursion to calculate powers, including handling of negative exponents, which extends the utility of the recursive approach. Employ these techniques in your Java applications to enhance their functionality and performance with recursive problem-solving strategies.
No comments yet.