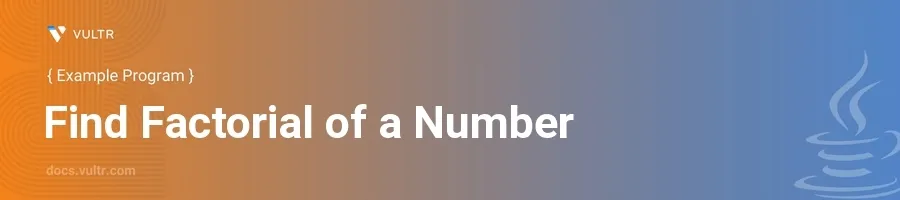
Introduction
The factorial of a non-negative integer ( n ) is the product of all positive integers less than or equal to ( n ). This concept is used frequently in permutations, combinations, and probability calculations. It's also a common example when learning programming languages due to its recursive nature and how it helps grasp the concept of recursion alongside iterative solutions.
In this article, you will learn how to calculate the factorial of a number in Java using both iterative and recursive methods. Explore the implementation of each method through detailed examples that ensure you can adapt these solutions in different programming scenarios.
Iterative Method for Calculating Factorial
Understanding the Iterative Approach
Start with a
for
loop that runs from ( 1 ) to ( n ).Initialize a result variable to ( 1 ) and multiply it by each number in the loop to get the factorial.
javapublic static int factorialIterative(int n) { int result = 1; for (int i = 1; i <= n; i++) { result *= i; } return result; }
The method
factorialIterative
multiplies theresult
by every integer from ( 1 ) to ( n ). This is a direct way to compute the factorial and is easy to understand for anyone learning loops and conditionals in Java.
Example of Iterative Factorial Calculation
Apply the method
factorialIterative
to compute the factorial of ( 5 ).javapublic static void main(String[] args) { int number = 5; System.out.println("Factorial of " + number + " is: " + factorialIterative(number)); }
When you execute this code, it will print the factorial of ( 5 ), which is ( 120 ).
Recursive Method for Calculating Factorial
Understanding the Recursive Approach
Create a method that calls itself with decremented values of ( n ) until ( n ) equals ( 1 ).
The recursion stops when ( n ) is ( 1 ), and at this point, the method begins to return and multiply the values together.
javapublic static int factorialRecursive(int n) { if (n == 1) { return 1; } else { return n * factorialRecursive(n - 1); } }
The method
factorialRecursive
calls itself with ( n-1 ) until ( n ) reaches ( 1 ). This implementation is a classical example of recursion in computer programming and helps understand the stack mechanism intuitively.
Example of Recursive Factorial Calculation
Execute the
factorialRecursive
method to determine the factorial of ( 7 ).javapublic static void main(String[] args) { int number = 7; System.out.println("Factorial of " + number + " is: " + factorialRecursive(number)); }
Running this code segment, ( 5040 ) is outputted, representing the factorial of ( 7 ).
Conclusion
The calculation of a factorial in Java can be approached both iteratively and recursively, each serving particular needs. The iterative method is straightforward and often preferred for its simplicity and clarity. In contrast, the recursive method provides a deeper understanding of function calls and stack behaviors in programming. Depending on the situation and performance considerations, choose the appropriate method to implement factorial calculations in your Java projects. By mastering both techniques, you enhance your proficiency in using Java for various mathematical and logical operations.
No comments yet.