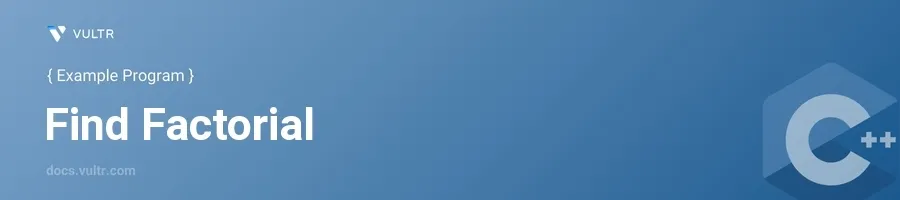
Introduction
Factorials are fundamental in various mathematical calculations, particularly in permutations, combinations, and algebraic functions. In C++, you can compute the factorial of a number using different methods such as iterative approach, recursive function, or leveraging standard libraries for handling large numbers.
In this article, you will learn how to implement factorial calculation in C++ through multiple examples. Discover how to use both iterative and recursive methods, and explore the impact of different approaches on performance and code complexity.
Calculating Factorial Iteratively
Factorial Using a Loop
Define a function that takes an integer as input.
Initialize the result to one inside the function.
Use a loop to multiply the result by each number up to the input number.
Return the result.
cpp#include <iostream> using namespace std; unsigned long long factorialIterative(int n) { unsigned long long result = 1; for (int i = 1; i <= n; i++) { result *= i; } return result; } int main() { int number = 5; cout << "Factorial of " << number << " is " << factorialIterative(number) << endl; return 0; }
In this code snippet, the function
factorialIterative
calculates the factorial of an integern
using a for loop. The result is printed in the main function.
Calculating Factorial Recursively
Recursive Function for Factorial
Define a recursive function to compute the factorial.
Check if the input is less than or equal to 1. If true, return 1.
Recursively multiply the number by the factorial of the number minus one.
Return the result from the recursive calls.
cpp#include <iostream> using namespace std; unsigned long long factorialRecursive(int n) { if (n <= 1) return 1; else return n * factorialRecursive(n - 1); } int main() { int number = 5; cout << "Factorial of " << number << " is " << factorialRecursive(number) << endl; return 0; }
The function
factorialRecursive
calculates the factorial using recursion. The base case is whenn
is less than or equal to 1, during which it returns 1. For other cases, it calculates the number multiplied by the factorial of the number minus one.
Conclusion
The factorial of a number can be computed in C++ using either an iterative or recursive approach. The iterative method involves using a loop and is generally more efficient and straightforward for large numbers. On the other hand, the recursive method provides a more mathematical and elegant approach, though it can lead to stack overflow with very large inputs. Depending on the situation, choose the appropriate method to integrate factorial calculations into your C++ programs effectively.
No comments yet.