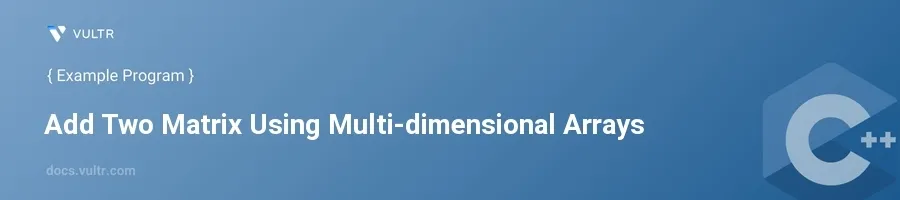
Introduction
Matrix operations are fundamental in various computational applications ranging from basic arithmetic to complex physics simulations. In C++, matrices can be efficiently handled using multi-dimensional arrays, allowing for operations such as addition, subtraction, and multiplication.
In this article, you will learn how to add two matrices using multi-dimensional arrays in C++. You will explore examples that demonstrate initializing matrices, performing addition, and displaying the results. This will help you grasp the practical application of matrix operations in C++ programming.
Adding Two Matrices
Define the Matrices
Start by declaring two two-dimensional arrays to hold the values of the matrices.
Initialize these arrays with the values for matrix addition.
cppint matrix1[2][2] = {{1, 2}, {3, 4}}; int matrix2[2][2] = {{5, 6}, {7, 8}};
These arrays represent two matrices of size 2x2. Each element in these matrices can be accessed using its row and column indices.
Perform Matrix Addition
Create another two-dimensional array to store the result of the addition.
Use nested loops to traverse each element in the matrices and perform addition.
cppint resultMatrix[2][2]; for (int i = 0; i < 2; i++) { for (int j = 0; j < 2; j++) { resultMatrix[i][j] = matrix1[i][j] + matrix2[i][j]; } }
This loop iterates over each row and column, adding corresponding elements from
matrix1
andmatrix2
and storing the result inresultMatrix
.
Display the Result
Use nested loops again to display the results stored in the resultant matrix.
cppstd::cout << "Resultant Matrix:" << std::endl; for (int i = 0; i < 2; i++) { for (int j = 0; j < 2; j++) { std::cout << resultMatrix[i][j] << " "; } std::cout << std::endl; }
This segment of code prints out each element of the resultant matrix, ensuring elements of the same row are printed on the same line followed by a new line for each row.
Conclusion
Adding two matrices using multi-dimensional arrays in C++ is a straightforward process that involves initializing arrays, employing nested loops for arithmetic operations, and displaying the results. This exercise not only enhances understanding of arrays and loops but also lays the groundwork for more complex matrix operations, which are vital in many scientific and engineering applications. Through these examples, develop proficiency in handling basic matrix arithmetic that forms the core of many advanced algorithms and applications in C++.
No comments yet.