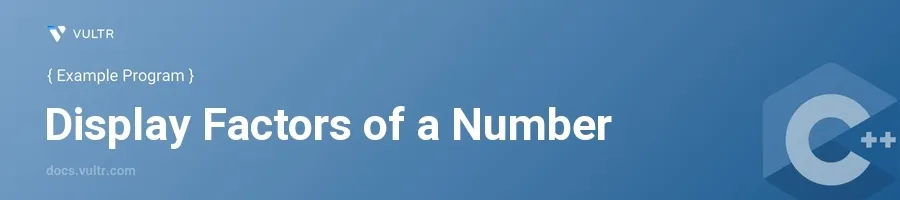
Introduction
Factors of a number are integers that can evenly divide the given number without leaving a remainder. In C++, calculating and displaying factors is a fundamental task that can help in understanding both loops and conditional statements better. This task also finds practical application in algorithms related to number theory, such as finding prime numbers or the greatest common divisor.
In this article, you will learn how to create a C++ program that displays all the factors of a given number. You'll see examples demonstrating how to implement this using simple loops and conditional statements, making it applicable even for those new to programming in C++.
Implementing the Factor Finder
Setup the Environment
Begin with a basic C++ program structure, including necessary headers and the main function.
cpp#include <iostream> using namespace std; int main() { // Code will go here return 0; }
This code includes the
iostream
library to handle input/output operations and uses the standard namespace.
Accept User Input
Declare a variable to store the number for which factors are to be found.
Prompt the user to enter a number and store the input.
cppint main() { int number; cout << "Enter a number: "; cin >> number; // Continuing the code }
Here,
number
will hold the value entered by the user, which will later be used to find its factors.
Calculate and Display Factors
Use a loop to iterate through possible divisors and check if they are factors.
Inside the loop, implement a condition to determine if each number is a factor.
Print each factor using standard output.
cppint main() { int number; cout << "Enter a number: "; cin >> number; cout << "Factors of " << number << " are: "; for(int i = 1; i <= number; ++i) { if (number % i == 0) { cout << i << " "; } } }
In this section, the loop iterates through all integers from 1 to
number
. The conditionalif (number % i == 0)
checks ifi
is a factor by verifying that the remainder of the division ofnumber
byi
is zero. If true, it printsi
as a factor.
Conclusion
By following these steps, you effortlessly set up a C++ program to find and display all factors of a given number. This example serves as a good practice for understanding loops and conditionals in C++, and it can also be extended or modified for various related applications, such as checking for prime numbers by counting the total number of factors. Adapt and integrate this simple yet effective technique into larger projects to handle more complex tasks involving number theory and arithmetic operations.
No comments yet.