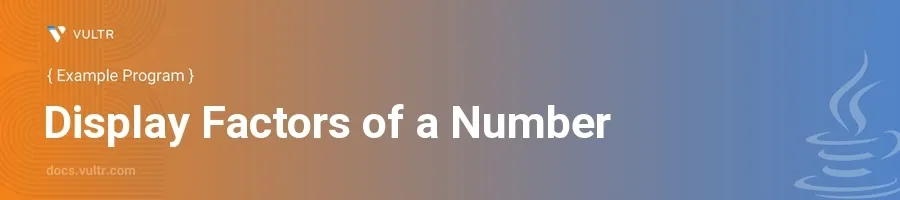
Introduction
Java is a versatile programming language often used for developing applications that range from mobile apps to large web systems. One common exercise for beginners is writing a program to display factors of a number, which enhances understanding of loops and conditional statements.
In this article, you will learn how to create a Java program to find and display the factors of a number. The examples provided will guide you through different scenarios, enhancing your ability to handle basic programming constructs such as loops and conditional logic in Java.
Understanding Factors of a Number
A factor of a number refers to any integer which divides that number without leaving a remainder. For instance, the factors of 12 are 1, 2, 3, 4, 6, and 12. This concept is integral in various mathematical computations and is also a cornerstone for certain algorithms in computer science.
Simple Java Program to Find Factors
Begin by setting up a basic Java program structure.
Initialize a variable to store the number whose factors are to be found.
Use a
for
loop to iterate through potential divisors.Use an
if
statement within the loop to check if the modulus operation yields zero, indicating a factor.javapublic class FactorFinder { public static void main(String[] args) { int number = 60; // Number to find the factors of System.out.println("Factors of " + number + " are:"); for(int i = 1; i <= number; ++i) { if(number % i == 0) { System.out.println(i); } } } }
Here, the program iterates from 1 to the number itself. Whenever the number is divisible by the iterator (
i
), that value ofi
is printed as a factor.
Enhancing the Program for Efficiency
Recognize that factors come in pairs.
Optimize by iterating only up to the square root of the number.
Print both factors in each pair unless the number is a perfect square.
javapublic class EfficientFactorFinder { public static void main(String[] args) { int number = 60; // Number to find the factors of System.out.println("Factors of " + number + " are:"); for(int i = 1; i <= Math.sqrt(number); ++i) { if(number % i == 0) { if (i != number / i) { System.out.println(i + ", " + (number / i)); } else { System.out.println(i); } } } } }
This version of the program reduces the number of iterations needed to find factors by checking only up to the square root of the number. For non-square numbers, it prints both factors discovered in each iteration.
Conclusion
Developing a Java program to display the factors of a number provides fundamental insights into the use of loops and conditionals in programming. Start with a simple approach to understand the logic and then optimize the program to enhance its performance and efficiency. By implementing these examples, ensure your foundational Java skills are solidified, paving the way for more complex programming tasks. Enjoy the process of learning and experimenting with Java, an essential language in the realm of software development.
No comments yet.