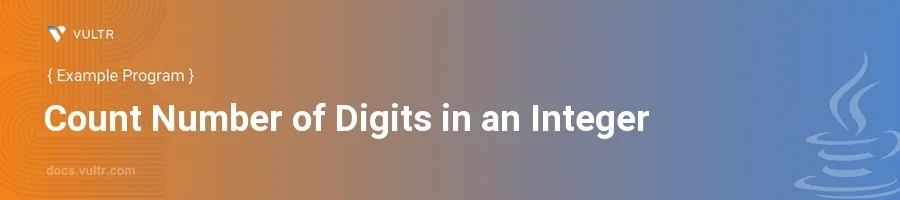
Introduction
When programming in Java, counting the number of digits in an integer is a common task that might seem trivial yet plays a crucial role in many applications such as digital signal processing, statistical analysis, or data validation tasks. This operation involves determining how many digits make up an integer, which can be achieved through various techniques.
In this article, you will learn how to count the digits of an integer in Java. These methods will range from using basic loops to leveraging more advanced Java built-in functions. Understanding these different approaches helps in picking the right tool for both simple and complex tasks.
Using Loops to Count Digits
Count Digits with a While Loop
Initialize a counter variable to store the count of digits.
Use a while loop to divide the number by 10 until it becomes 0.
javapublic class DigitCounter { public static int countDigits(int number) { int count = 0; while (number != 0) { number /= 10; count++; } return count; } }
This code defines a method
countDigits
which takes an integernumber
as its parameter. The while loop runs as long asnumber
is not zero, dividing the number by 10 in each iteration, which effectively removes the last digit of the number.count
is incremented in each iteration to keep track of the number of digits.
Count Digits with a For Loop
Convert the integer to a string and use a for loop to iterate over its characters.
javapublic class DigitCounter { public static int countDigits(int number) { String numberAsString = Integer.toString(number); int count = 0; for (int i = 0; i < numberAsString.length(); i++) { count++; } return count; } }
This method converts the integer
number
to a string. It then initializes a countercount
and iterates over each character in the string. The counter is incremented with each iteration, resulting in the total digit count by the end of the loop.
Using Java Built-in Functions
Using String Conversion
Convert the integer to a string and use its length property.
javapublic class DigitCounter { public static int countDigits(int number) { return Integer.toString(number).length(); } }
Here, the integer is converted into a string using
Integer.toString(number)
, and the length of this string directly represents the number of digits. This one-liner is an efficient way to find the digit count without looping.
Conclusion
Counting the number of digits in an integer in Java can be approached in several ways—from iterative loops to utilizing built-in string functions. Each method has its use depending on the circumstance and required efficiency of the code. Use loops for educational purposes or when handling integers as numeric values directly, and consider the string conversion approach for concise, efficient code. Employ these techniques in various Java applications to enhance your programming capabilities, ensuring reliability and efficiency in digit counting tasks.
No comments yet.