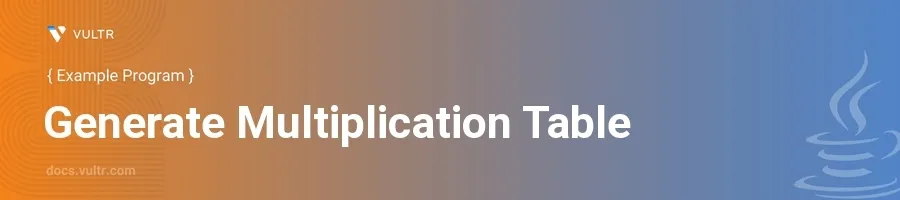
Introduction
Generating a multiplication table is a common task in many introductory programming courses and exercises. It involves creating a systematic arrangement of numbers where each row and column represent sequential multipliers of a base number. In Java, this can be achieved using loops and simple arithmetic operations.
In this article, you will learn how to create a multiplication table in Java. Techniques for both console output and graphical representation using Swing will be detailed. By the end, you'll understand how to implement loops effectively for this purpose and how to display the results in different formats.
Generating a Multiplication Table to the Console
Basic Console Output
Start with the basics by creating a Java program that prints a multiplication table to the console.
Define the size of the table and the number to generate the table for using nested loops.
javapublic class MultiplicationTable { public static void main(String[] args) { int number = 5; // Multiplication table for 5 int size = 10; // Display from 1 to 10 for (int i = 1; i <= size; i++) { System.out.printf("%d * %d = %d\n", number, i, number * i); } } }
This program will output the multiplication table for 5 up to 10. The loop iterates through the numbers 1 to 10, multiplying each by 5 and printing the result in a formatted way.
Flexible User Input
Enhance the program by allowing user input to specify both the number and the size of the table.
Use
Scanner
to get input from the console.javaimport java.util.Scanner; public class MultiplicationTable { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the number for the multiplication table: "); int number = scanner.nextInt(); System.out.print("Enter the maximum multiplier: "); int size = scanner.nextInt(); for (int i = 1; i <= size; i++) { System.out.printf("%d * %d = %d\n", number, i, number * i); } scanner.close(); } }
In this version, the user can enter any number and any size for the multiplication table. The
Scanner
class is used to capture input from the console.
Generating a Multiplication Table Using Java Swing
Basic Swing Application
Create a simple GUI application that displays a multiplication table in a more visually appealing format.
Use
JTable
within aJFrame
to arrange and display the multiplication results.javaimport javax.swing.*; import javax.swing.table.DefaultTableModel; public class MultiplicationTableGUI { public static void main(String[] args) { SwingUtilities.invokeLater(() -> createAndShowGUI()); } private static void createAndShowGUI() { JFrame frame = new JFrame("Multiplication Table"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(500, 200); String[] columnNames = {"Multiplier", "Result"}; String[][] data = new String[10][2]; int number = 5; // Multiplication table for 5 for (int i = 0; i < 10; i++) { data[i][0] = "5 * " + (i+1); data[i][1] = String.valueOf(5 * (i+1)); } JTable table = new JTable(data, columnNames); JScrollPane scrollPane = new JScrollPane(table); frame.add(scrollPane); frame.setVisible(true); } }
This example sets up a basic Swing window with a table displaying the multiplication table for the number 5. The table is populated within the GUI initialization, and the results are provided in a scrollable pane.
Conclusion
Creating a multiplication table in Java demonstrates fundamental programming techniques such as loops and input/output operations. You explored how to implement these both for console applications and graphical interfaces using Swing. Apply these concepts to enhance your understanding of Java programming, improving both your coding skills and your ability to visualize data results effectively. Use the basic console methods for quick experiments or the Swing approach for more user-friendly applications.
No comments yet.