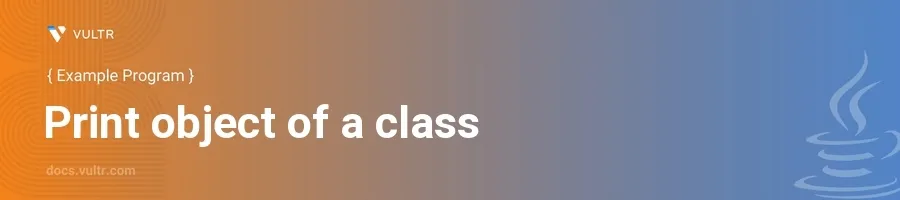
Introduction
Java is widely used for its powerful object-oriented capabilities, including class definition, object creation, and method invocation. A common task in Java programming is to print an object's state, which can be achieved by overriding the toString()
method in a class. This allows for a more readable and informative output when an object is printed, rather than displaying the default hash code representation.
In this article, you will learn how to effectively print object details in Java by creating classes and overriding the toString()
method. You'll explore several examples that demonstrate how to customize the output to make debugging and logging more informative and useful.
Basic Printing of a Class Object
Override the toString()
Method
Define a simple class with some attributes.
Override the
toString()
method to customize the output when an object of the class is printed.javapublic class Person { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } @Override public String toString() { return "Person{name='" + name + "', age=" + age + '}'; } }
This example defines a
Person
class with propertiesname
andage
and overrides thetoString()
method to return a string that clearly displays the object's attributes.
Instantiate and Print the Object
Create an object of the
Person
class.Print the object using
System.out.println()
.javapublic class Main { public static void main(String[] args) { Person person = new Person("John Doe", 30); System.out.println(person); } }
When this code runs, it prints
Person{name='John Doe', age=30}
, thanks to the overriddentoString()
method.
Advanced Example with Complex Objects
Define a Class with Composite Attributes
Create a new class that includes various data types and possibly other objects as attributes.
Override
toString()
to provide a detailed representation of the object.javapublic class Employee { private String name; private int id; private Department department; public Employee(String name, int id, Department department) { this.name = name; this.id = id; this.department = department; } @Override public String toString() { return "Employee{" + "name='" + name + '\'' + ", id=" + id + ", department=" + department + '}'; } } public class Department { private String name; public Department(String name) { this.name = name; } @Override public String toString() { return "Department{name='" + name + "'}"; } }
This example includes an
Employee
class that has aDepartment
object as an attribute. Both classes override thetoString()
method for meaningful printing.
Instantiate and Print Composite Object
Create objects of
Employee
andDepartment
.Print the
Employee
object to see detailed output including theDepartment
information.javapublic class Main { public static void main(String[] args) { Department department = new Department("IT"); Employee employee = new Employee("Alice Johnson", 101, department); System.out.println(employee); } }
Here, the output will display all the attributes of
Employee
, including the nestedDepartment
details:Employee{name='Alice Johnson', id=101, department=Department{name='IT'}}
.
Conclusion
By overriding the toString()
method in Java classes, you gain control over how objects are represented as strings, which is extremely useful for monitoring and debugging purposes. You have seen how this can be applied to simple objects and to more complex scenarios involving nested objects. Implement these techniques in your Java applications to enhance the readability and maintainability of logging and output statements, ensuring that object states are clear and informative when printed.
No comments yet.