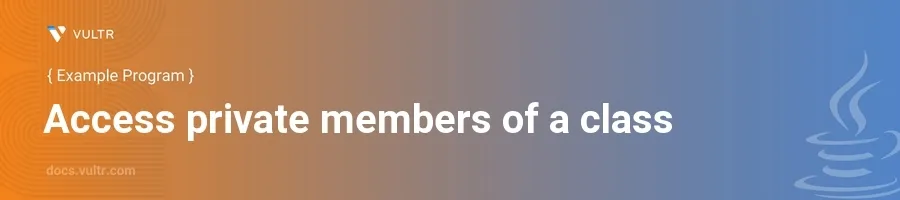
Introduction
Private members of a class in Java are crucial for encapsulating and safeguarding the data. These members are only accessible within the class they are declared and are hidden from other classes. This feature is fundamental in object-oriented programming as it helps maintain the integrity of the data by preventing external interference.
In this article, you will learn how to access private members of a class using various methods in Java. We will explore practical examples like using getters and setters, reflection, and through nested classes to demonstrate how you can interact with private fields and methods, despite their access restrictions.
Using Getters and Setters
Basic Approach to Access Private Fields
Define a class with private fields.
Create public getter and setter methods for these fields.
javapublic class Person { private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } }
In this example, the
Person
class has a private string member calledname
. ThegetName()
andsetName(String name)
methods are public, which makes it possible to get and set thename
from outside the class.
Example of Using Getters and Setters
Create an instance of the
Person
class.Use the setter and getter methods to access the private field.
javapublic class Main { public static void main(String[] args) { Person person = new Person(); person.setName("John"); System.out.println("Name: " + person.getName()); } }
Here, you create an object
person
of thePerson
class and use thesetName()
method to set the name as "John". Then, you retrieve the name usinggetName()
method and print it.
Using Reflection to Access Private Members
Introduction to Reflection
- Understand that Java Reflection API allows you to inspect and manipulate classes, interfaces, constructors, methods, and fields at runtime.
Accessing Private Fields Using Reflection
Obtain the
Class
object.Access the private field using the
getField
method.Make the field accessible by calling
setAccessible(true)
.javaimport java.lang.reflect.Field; public class Main { public static void main(String[] args) { Person person = new Person(); person.setName("John"); try { Field nameField = Person.class.getDeclaredField("name"); nameField.setAccessible(true); String nameValue = (String) nameField.get(person); System.out.println("Reflection Name: " + nameValue); } catch (Exception e) { e.printStackTrace(); } } }
In this code, you use reflection to access the private
name
field of thePerson
class. After obtaining the field, you callsetAccessible(true)
to override the access check, which lets you read the private field.
Access Through Nested Classes
Inner Classes and Private Access
- Realize that inner classes in Java can access all members of the outer class, including private fields.
Using an Inner Class to Access Private Members
Create an inner class that modifies the private fields of the outer class.
javapublic class OuterClass { private String secret = "Top Secret"; class InnerClass { void revealSecret() { System.out.println("The secret is: " + secret); } } public void displaySecret() { InnerClass inner = new InnerClass(); inner.revealSecret(); } }
Here,
OuterClass
has a private stringsecret
. The inner classInnerClass
has a methodrevealSecret()
that accesses the outer class's private fieldsecret
directly.
Example of Executing the Inner Class Method
Instantiate the outer class.
Call the method that uses the inner class to access the private member.
javapublic class Main { public static void main(String[] args) { OuterClass outer = new OuterClass(); outer.displaySecret(); } }
This code creates an instance of
OuterClass
and callsdisplaySecret()
. This method creates an instance of theInnerClass
and calls itsrevealSecret()
method, which accesses and prints the privatesecret
.
Conclusion
Accessing private members of a class in Java demonstrates the flexibility and capabilities of the language, even with strict encapsulation rules. While getters and setters are the standard for accessing private fields, techniques like reflection and use of inner classes offer powerful alternatives for special circumstances. Each method has its suitable use case, and understanding when to employ each technique ensures your code remains robust and maintainable. Embrace these methods to enhance your Java programming skills and to handle class data effectively.
No comments yet.