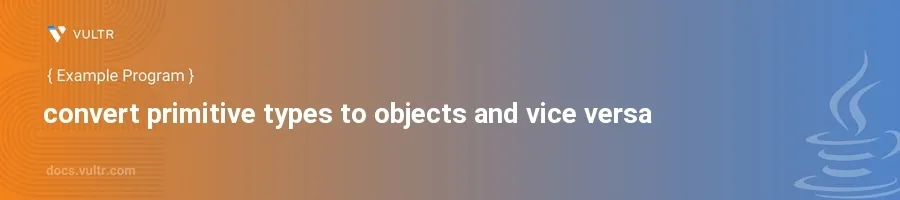
Introduction
Java programming supports both primitive data types and their corresponding wrapper classes, which allow primitives to be used as objects. This functionality is crucial for utilizing frameworks and collections that work exclusively with objects. Converting between primitive types and their respective object wrappers is an essential skill for Java developers.
In this article, you will learn how to convert primitive data types to their corresponding object forms and vice versa in Java. Through a set of practical examples, explore the automatic and manual conversions enabled by Java's features such as autoboxing and unboxing.
Conversion Basics
Autoboxing: Primitive to Object
Autoboxing is the automatic conversion that the Java compiler makes between the primitive types and their corresponding object wrapper classes.
Declare a primitive data type.
Assign the primitive to a wrapper class reference.
javaint primInt = 100; Integer objInt = primInt;
The primitive
int
value100
is automatically converted into anInteger
object.
Unboxing: Object to Primitive
Unboxing is the reverse process of autoboxing. It is the automatic conversion of a wrapper class to its corresponding primitive type.
Define a wrapper class object.
Assign it to a primitive data type.
javaInteger objInteger = new Integer(100); int primitiveInt = objInteger;
Here, the
Integer
object is automatically converted to the primitiveint
.
Manual Conversion Techniques
Using .intValue()
and Similar Methods
Sometimes it is preferable to perform conversions manually, especially to make the code intentions explicit or to control when conversions happen.
Use
.intValue()
for convertingInteger
toint
.Use similar methods for other data types.
javaInteger objInteger = Integer.valueOf(500); int manualInt = objInteger.intValue(); Double objDouble = Double.valueOf(3.14); double manualDouble = objDouble.doubleValue();
In the examples above,
.intValue()
and.doubleValue()
are explicitly called to convert wrapper objects to their respective primitives.
Using valueOf()
for Object Creation
To ensure precise control over object creation, especially in the context of boxing, valueOf()
is commonly used.
Convert primitive data to objects using
valueOf()
.This avoids the creation of unnecessary objects unlike constructors deprecated in newer Java versions.
javaint someInt = 300; Integer objFromInt = Integer.valueOf(someInt); double someDouble = 45.67; Double objFromDouble = Double.valueOf(someDouble);
valueOf()
is used to create instances of wrapper classes from primitive types, which is preferred over using constructors for this purpose.
Working with Other Primitive Types
Boolean and Character
Both Boolean
and Character
classes provide mechanisms for boxing and unboxing that are similar to Integer
and Double
.
Autoboxing and unboxing for boolean and char types.
javachar primChar = 'a'; Character objChar = primChar; // Autoboxing char backToPrimitive = objChar; // Unboxing boolean primBoolean = true; Boolean objBoolean = primBoolean; // Autoboxing boolean backToPrim = objBoolean; // Unboxing
Conversion Involving Arrays
Arrays of primitives and their corresponding wrapper classes can also be converted, although not automatically.
Convert an array of primitives to an array of objects using a loop or a utility method.
Convert back by iterating through the array or using Java 8's streams for more compact code.
javaint[] primitiveArray = {1, 2, 3, 4}; Integer[] objectArray = new Integer[primitiveArray.length]; // Converting to objects for (int i = 0; i < primitiveArray.length; i++) { objectArray[i] = Integer.valueOf(primitiveArray[i]); } // Converting back to primitives int[] backToPrimitiveArray = new int[objectArray.length]; for (int i = 0; i < objectArray.length; i++) { backToPrimitiveArray[i] = objectArray[i]; }
In these examples, manual looping converts arrays from primitives to objects and vice versa. Java 8 Streams can also be used for a more modern and less verbose approach.
Conclusion
Understanding and utilizing the conversion between primitive types and object wrappers in Java is critical for engaging with object-oriented features and APIs that require objects. Whether using automatic features like autoboxing and unboxing or performing manual conversions using methods like .intValue()
or valueOf()
, Java provides sufficient flexibility to manage these transformations efficiently. By mastering these conversions, Java applications can be optimized for both performance and interoperability within various frameworks and libraries.
No comments yet.