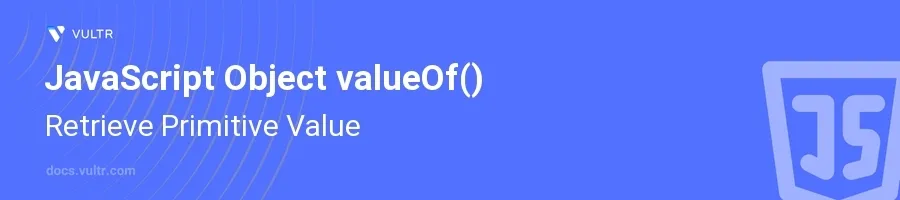
Introduction
The valueOf()
method in JavaScript is an essential function for object manipulation, primarily when you work with customized or built-in objects that need to reflect a specific, primitive value. When JavaScript expects a primitive value from an object, the valueOf()
method is automatically called to convert an object to a primitive value, which could be a number, string, or boolean value depending on the context or how it’s defined in the object.
In this article, you will learn how to harness the valueOf()
method effectively across various objects and situations. Explore practical implementations to override the default method to customize object behavior in your JavaScript code.
Understanding valueOf() in JavaScript
Default Behavior
Understand that all objects in JavaScript inherit
valueOf()
fromObject.prototype
.Recognize that the default
valueOf()
method does not always need to be overridden, as it returns the object itself if not specifically defined.javascriptconst obj = {}; console.log(obj.valueOf() === obj); // prints true
This example verifies that the default
valueOf()
returns the object itself sinceobj
does not definevalueOf()
explicitly.
Custom Implementation of valueOf()
Define a custom object and override the
valueOf()
method to provide meaningful primitive output.javascriptfunction MyNumberType(n) { this.number = n; } MyNumberType.prototype.valueOf = function() { return this.number; }; const objectInstance = new MyNumberType(5); console.log(objectInstance + 5); // prints 10
In this snippet,
MyNumberType
function creates an object which has a propertynumber
. ThevalueOf()
method is overridden to return thenumber
property hence allowing primitive operations like addition.
valueOf() in Date Objects
Revisit Date objects which inherently have a meaningful
valueOf()
that returns the timestamp.javascriptconst now = new Date(); console.log(now.valueOf()); // prints a numeric timestamp
Here,
valueOf()
for a Date object retrieves the timestamp, demonstrating a practical usage ofvalueOf()
in built-in JavaScript types.
Using valueOf() with Logical Operations
Simplify Logical Checks
Use
valueOf()
to simplify conditions in logical operations.javascriptfunction BooleanType(value) { this.value = value; } BooleanType.prototype.valueOf = function() { return !!this.value; // Convert this.value to a boolean }; const trueObject = new BooleanType(true); if (trueObject) { console.log('True!'); // prints 'True!' }
In this example,
valueOf()
is used to convert an object to an essential Boolean, streamlining its usability in conditional structures likeif
statements.
Conclusion
The valueOf()
method in JavaScript provides powerful functionality for manipulating object behavior within your programs. With the ability to customize this method, tailor how your objects interact in expressions and logical operations, ensuring your code operates as intended with optimal readability and efficiency. Through mastering valueOf()
, elevate your JavaScript coding skillset, allowing for richer, more intuitive code interactions.
No comments yet.