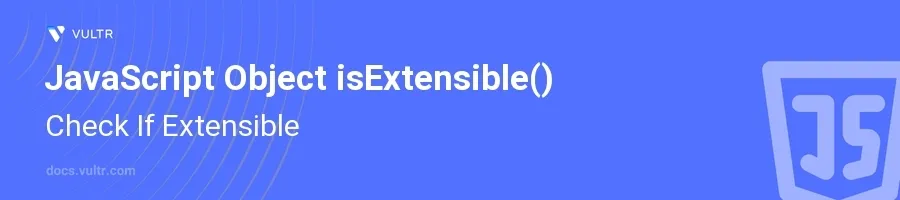
Introduction
JavaScript's Object.isExtensible()
method is a fundamental part of working with objects in JavaScript, particularly useful in managing and securing object properties. This method determines if new properties can be added to an object, which is crucial when enforcing immutability or working with sealed or frozen objects.
In this article, you will learn how to use the Object.isExtensible()
method effectively. You will explore examples demonstrating how to check if an object is extensible, and how the extensibility of an object changes with methods like Object.preventExtensions()
, Object.seal()
, and Object.freeze()
.
Understanding Object.isExtensible()
Basic Usage of isExtensible()
Create an object.
Use
Object.isExtensible()
to check if the object is extensible.javascriptvar obj = {}; var extensible = Object.isExtensible(obj); console.log(extensible); // Output: true
In this code,
Object.isExtensible(obj)
checks if it's possible to add new properties toobj
. As a result, it logstrue
, indicating the object is extensible.
Behavior After Using Object.preventExtensions()
Apply
Object.preventExtensions()
to an object.Recheck its extensibility.
javascriptvar fixedObj = {}; Object.preventExtensions(fixedObj); var isExtensibleNow = Object.isExtensible(fixedObj); console.log(isExtensibleNow); // Output: false
This snippet makes
fixedObj
non-extensible usingObject.preventExtensions()
. When checked withObject.isExtensible()
, it returnsfalse
, confirming that no new properties can be added.
Comparing with Object.seal() and Object.freeze()
Understand that both
Object.seal()
andObject.freeze()
also prevent adding new properties.Test extensibility on objects processed by these methods.
javascriptvar sealedObj = {}; Object.seal(sealedObj); console.log(Object.isExtensible(sealedObj)); // Output: false var frozenObj = {}; Object.freeze(frozenObj); console.log(Object.isExtensible(frozenObj)); // Output: false
Here, both
sealedObj
andfrozenObj
are checked for extensibility. The methodsObject.seal()
andObject.freeze()
not only prevent new properties from being added but also set the extensibility tofalse
.
Conclusion
The Object.isExtensible()
function in JavaScript is pivotal for understanding and controlling the modifiability of objects in your programming projects. By recognizing when and how to control an object's extensibility, you enhance the integrity and security of your application. Whether it's for ensuring that your data structures are immutable or for enforcing strict object property management, knowing how to check and set object extensibility is a skill that can significantly improve your coding practices.
No comments yet.