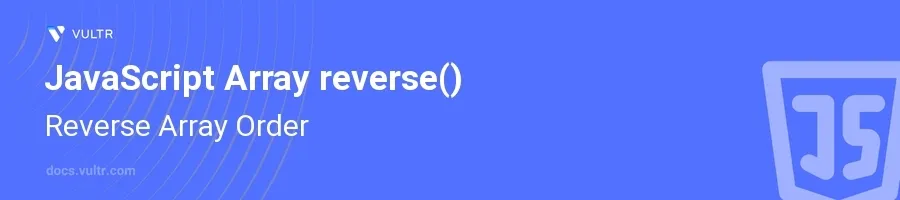
Introduction
The reverse()
method in JavaScript is a straightforward and powerful function used to reverse the order of elements within an array. This method modifies the array in place and returns the same array with its elements reversed, making it a useful tool in various programming tasks, such as algorithm development and data processing.
In this article, you will learn how to use the reverse()
method to invert the order of array elements. Explore practical examples that demonstrate how to reverse arrays containing different data types, and understand how this method affects the original array.
Reversing an Array of Numbers
Simple Array Reversal
Create an array of numbers.
Apply the
reverse()
method.javascriptlet numberArray = [1, 2, 3, 4, 5]; numberArray.reverse(); console.log(numberArray);
This code snippet reverses the
numberArray
. The console output will be[5, 4, 3, 2, 1]
showing the array elements in reverse order.
Reversing and Using the Reversed Array
Consider using the reversed array for further operations.
Perform a calculation or manipulation using the reversed array.
javascriptlet prices = [4.25, 5.99, 3.50, 7.15]; let reversedPrices = prices.reverse(); let taxIncludedPrices = reversedPrices.map(price => price * 1.2); console.log(taxIncludedPrices);
After reversing, this snippet maps over the
reversedPrices
to include tax. The manipulation demonstrates using the reversed array directly in subsequent calculations.
Reversing Arrays Containing Strings
Basic String Array Reversal
Initialize an array with string elements.
Use the
reverse()
method.javascriptlet names = ["Alice", "Bob", "Carol", "David"]; names.reverse(); console.log(names);
This outputs the array
names
in reverse order:["David", "Carol", "Bob", "Alice"]
.
Combining with Other Array Methods
Combine
reverse()
with other array methods likesort()
.First, sort the array, then reverse it.
javascriptlet fruits = ["banana", "apple", "orange", "mango"]; fruits.sort().reverse(); console.log(fruits);
This snippet sorts the array alphabetically and then reverses it. The final output is
["orange", "mango", "banana", "apple"]
, demonstrating how to chainreverse()
withsort()
.
Implications of Reversing Arrays
It's important to remember that reverse()
changes the original array itself. Here's what you need to consider:
The original array is mutated—this means that any other references to the original array also reflect the reversed changes.
To prevent mutation of the original array, you can first make a copy of the array and then apply
reverse()
.javascriptlet original = [1, 2, 3, 4, 5]; let reversedCopy = [...original].reverse(); console.log("Original:", original); console.log("Reversed Copy:", reversedCopy);
This will show that the
original
array remains unchanged, whilereversedCopy
contains the reversed elements.
Conclusion
The reverse()
method in JavaScript is a convenient and efficient way to reverse the elements of an array. While extremely useful in many scenarios, always consider the direct modification it makes to the original array. With the examples provided, effectively reverse arrays containing different data types and integrate this functionality into broader data processing and manipulation tasks. Apply these concepts to keep your code clean and effective, enhancing both readability and performance.
No comments yet.