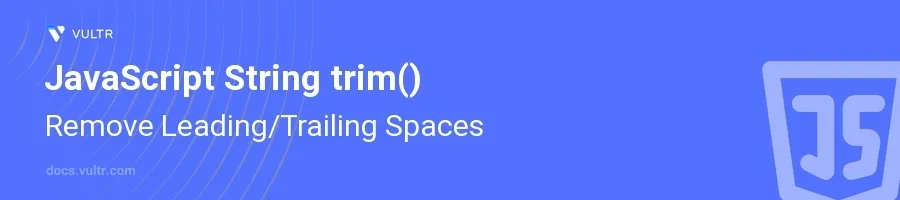
Introduction
The trim()
method in JavaScript is a convenient and frequently used function for removing whitespace from both ends of a string. This functionality is pivotal in data cleaning processes such as preparing user inputs for processing or storage, ensuring consistency, and avoiding errors during comparisons or validations.
In this article, you will learn how to apply the trim()
method to sanitize string inputs effectively. Explore practical applications and subtle nuances of this method to enhance your JavaScript programming skills and data handling capabilities.
Understanding the trim() Method
Basic Usage of trim()
Start with a basic string that includes unwanted spaces at the beginning and the end.
Apply the
trim()
method to remove these spaces.javascriptlet greeting = ' Hello world! '; let trimmedGreeting = greeting.trim(); console.log(trimmedGreeting);
This example demonstrates how
trim()
is used to clean up 'greeting'. The resulting output is 'Hello world!' without the leading and trailing spaces.
Handling Tab and Newline Characters
Recognize that
trim()
also removes tab, carriage return, and newline characters from the start and end of the string.Use a string that contains these types of whitespace characters.
Apply the
trim()
method to see the effect on such characters.javascriptlet messyString = '\t\n Hello world! \n\t'; let cleanString = messyString.trim(); console.log(cleanString);
Here,
trim()
eliminates all tab (\t
) and newline (\n
) characters from both ends, leaving only 'Hello world!'.
Practical Applications of trim()
Validating Form Inputs
Assume handling user input from a form where spaces in input fields can lead to validation errors or incorrect data processing.
Use
trim()
to clean the data before performing any checks or operations.javascriptfunction validateInput(input) { let trimmedInput = input.trim(); if (trimmedInput === '') { console.log('Input is required'); } else { console.log('Input is valid'); } } validateInput(' '); // Outputs: Input is required validateInput(' John Doe '); // Outputs: Input is valid
The function
validateInput
trims the input and checks if it is empty, providing appropriate feedback based on whether the user has entered meaningful content.
Integrating with Other String Methods
Combine
trim()
with other String methods to perform complex manipulations.Implement a scenario where a formatted output of a user's full name is required.
javascriptlet fullName = ' Jane Doe '; let formattedName = fullName.trim().replace(/\s+/g, ' '); console.log(formattedName);
After trimming the whitespace, the
replace
method is used to convert multiple spaces between names into a single space, yielding 'Jane Doe'.
Conclusion
The trim()
method is an essential tool in JavaScript for maintaining clean, user-friendly data handling by removing unwanted spaces, tabs, and newline characters from strings. Its simplicity allows it to be seamlessly integrated with other string operations, making it incredibly useful in scenarios involving form validations, user input sanitizations, and more. Leveraging trim()
, ensure data integrity and improve user interactions in your web applications.
No comments yet.