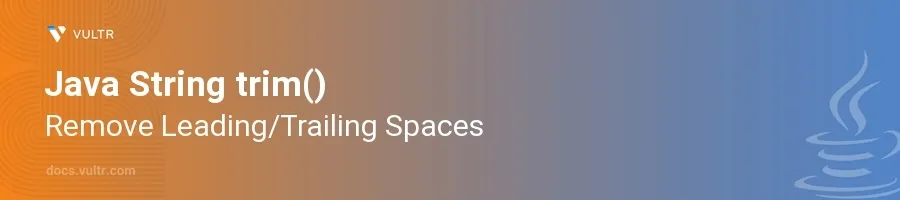
Introduction
The trim()
method in Java is crucial for removing unwanted leading and trailing whitespace from strings. This functionality is especially significant when processing user inputs or during text parsing where unnecessary spaces can lead to errors or misrepresented data. As whitespace can affect the logic of data comparisons and subsequent operations, ensuring string cleanliness is essential.
In this article, you will learn how to effectively use the trim()
method in Java to enhance data processing. Discover various scenarios where trimming strings becomes beneficial, and see practical code examples that demonstrate its usage.
Understanding the trim() Method
Basic Usage of trim()
Start with a basic string that includes both leading and trailing spaces.
Use the
trim()
method to remove these spaces.javaString original = " Hello, World! "; String trimmed = original.trim(); System.out.println(trimmed);
Here,
original
string contains spaces around the text. After applyingtrim()
, thetrimmed
string outputs "Hello, World!" without the extra spaces.
Verify the Effectiveness of trim()
Compare the lengths of the original and trimmed strings to see the effect of the
trim()
method.javaSystem.out.println("Original length: " + original.length()); System.out.println("Trimmed length: " + trimmed.length());
This code segment outputs the length of the strings before and after trimming, highlighting how
trim()
effectively reduces the string size by removing spaces.
Caution with trim()
Understand that
trim()
only removes spaces and other whitespace characters based on the Unicode definition of whitespace.Recognize that
trim()
does not affect spaces within the string.javaString internalSpaces = "Java Platform"; String trimmedString = internalSpaces.trim(); System.out.println(trimmedString);
Although the string "Java Platform" has multiple spaces between words,
trim()
will not remove these. It only removes from the ends, not between.
Practical Applications of trim()
Cleaning User Input
Trim user input to prepare for safe, accurate processing or storage.
Implement
trim()
in a login system where spaces could prevent proper username recognition.javaString usernameInput = " john_doe123 "; String cleanUsername = usernameInput.trim();
Trimming here helps in preventing logical errors such as mismatches due to invisible characters like spaces.
Data Parsing and Processing
Utilize
trim()
in data parsing, essential when data is imported from files that may contain extra padding spaces.Example: Parsing a comma-separated values (CSV) file with unwanted spaces around values.
javaString csvLine = "Steve, Apple, CEO "; String[] values = csvLine.trim().split(",");
Trimming before splitting ensures that the job title does not carry trailing spaces, which could affect further data manipulations or display.
Formatted Output
Use
trim()
to maintain the aesthetics and correctness of user-generated content and system messages.Example: Preparing a statement for display or printing.
javaString announcement = " New store opening soon! "; System.out.println(announcement.trim());
Trimming in this scenario ensures the public announcement appears neat and free from formatting errors when displayed.
Conclusion
The trim()
function in Java plays a pivotal role in the manipulation and handling of strings by removing unwanted leading and trailing whitespace. Not only does it improve the functionality and user experience by ensuring data is presented and processed correctly, but it also guards against common input errors. Leverage this method in various applications from user input sanitization, data parsing, to formatting outputs, ensuring your Java projects handle strings efficiently and effectively. Through the examples and scenarios discussed, embrace trim()
to enhance both the back-end logic and front-end display of your applications.
No comments yet.