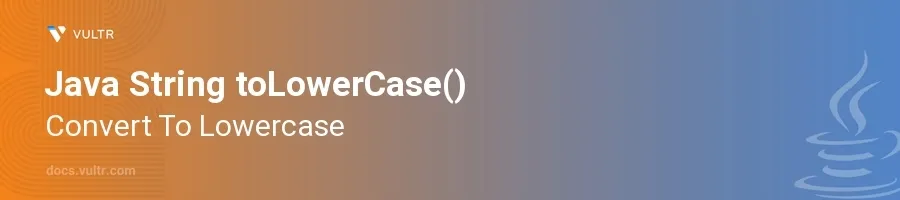
Introduction
The toLowerCase()
method in Java is part of the String class and is used to convert all characters in a given string to lowercase. This method is extremely handy when you need to normalize text for comparison or processing purposes. It helps in achieving case-insensitive user inputs and can be used to prepare data for sorting or indexing.
In this article, you will learn how to effectively utilize the toLowerCase()
method in Java. Explore various examples that demonstrate how this method works and understand how to apply it in real-world programming scenarios.
Understanding toLowerCase()
What is toLowerCase()?
- Recognize that
toLowerCase()
is a method from the Java String class. - Understand that it converts every character of the string to its lowercase equivalent based on rules of the default locale unless specified otherwise.
Default Locale Usage
Start with a basic usage of
toLowerCase()
which uses the system’s default locale implicitly.javaString statement = "Hello World"; String lowerCaseStatement = statement.toLowerCase(); System.out.println(lowerCaseStatement); // Output: hello world
In this code snippet, the string "Hello World" is converted to "hello world". Here, the system's default locale is used to determine how to handle locale-specific conversions.
Specific Locale Usage
Utilize
toLowerCase(Locale locale)
to explicitly specify a locale.Realize the importance of specifying a locale in multilingual applications where text needs to be converted in a locale-specific manner.
javaimport java.util.Locale; String germanStatement = "GRÜßEN"; String lowerCaseGerman = germanStatement.toLowerCase(Locale.GERMAN); System.out.println(lowerCaseGerman); // Output: grüßen
This example demonstrates converting "GRÜßEN" into lowercase using the German locale, where the uppercase 'ß' is properly converted to 'ß' itself since in German alphabet 'ß' remains the same in both uppercase and lowercase forms.
Practical Use Cases
Case-insensitive Comparisons
Apply
toLowerCase()
for making string comparisons regardless of the character case.Compare user input with predefined strings to handle commands or responses in a case-insensitive manner.
javaString userInput = "Exit"; String command = "exit"; if (userInput.toLowerCase().equals(command)) { System.out.println("User chose to exit."); } else { System.out.println("Unknown command."); }
In this scenario,
toLowerCase()
allows comparison of user input "Exit" with a standard "exit" command without case sensitivity, improving user experience and functionality efficiency.
Normalizing Data
Use
toLowerCase()
to normalize text data before processing.Normalize user-generated content or textual data retrieved from different sources for consistency.
javaString rawInput = "Email@Example.COM"; String normalizedEmail = rawInput.toLowerCase(); System.out.println(normalizedEmail); // Output: email@example.com
Here, converting the email address to all lowercase ensures uniform format, especially useful in databases and data processing scenarios where consistency is key.
Conclusion
The toLowerCase()
function in Java serves as a straightforward yet powerful tool for text manipulation, ensuring that strings can be converted to lowercase efficiently and consistently. This capability is fundamental in creating applications that handle text input in a user-friendly and error-free manner, whether it's for simple commands or complex multilingual content. Proper understanding and implementation of this method enhance data processing, facilitate robust data comparison, and maintain data integrity in software development. By integrating the examples provided, you empower your Java applications to handle text in a more flexible and error-resistant manner.
No comments yet.