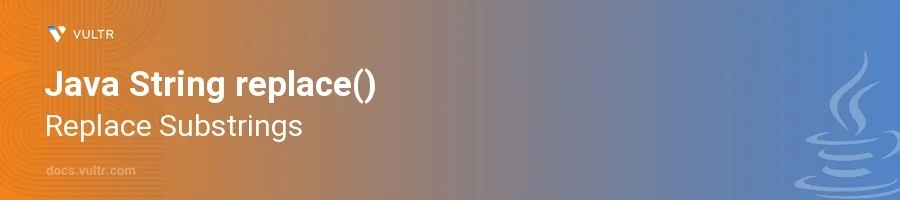
Introduction
The replace()
method in Java is an essential tool for string manipulation, allowing developers to replace occurrences of a substring or character within a string with a new substring or character. This functionality is crucial for data cleaning, modifying content, and improving the readability of text output in Java applications.
In this article, you will learn how to effectively use the replace()
method in various scenarios. Discover the intricacies of replacing characters and substrings in a string, how this method handles different data types, and explore practical examples that you can apply directly in your own Java projects.
Understanding the replace() Method
Basic Usage of replace()
Import the Java package containing the
String
class. This step is typically covered implicitly by the Java environment sinceString
is a fundamental data type.Initialize a string variable with content.
Apply the
replace()
method to swap out certain characters or substrings.javaString original = "Hello World"; String modified = original.replace("World", "Java"); System.out.println(modified);
Here,
"World"
in the stringoriginal
is replaced with"Java"
. Consequently,modified
will outputHello Java
.
Case Sensitivity
Remember that
replace()
is case-sensitive.Create an example where case sensitivity matters.
javaString greeting = "Hello World"; String changedGreeting = greeting.replace("world", "Java"); System.out.println(changedGreeting);
This code tries to replace
"world"
with"Java"
. However, because"world"
does not match the case of"World"
, the original string remains unchanged, and soHello World
is printed instead of changing it.
Replacing Characters
Single Character Replacement
Consider a scenario where only single characters need to be replaced.
Utilize the
replace()
method for replacing single characters.javaString example = "Java"; String updatedExample = example.replace('a', 'o'); System.out.println(updatedExample);
Every instance of
'a'
in"Java"
has been replaced with'o'
, resulting in the outputJovo
.
Replacing Special Characters
Identify any special or non-alphanumeric characters in the string.
Use the
replace()
method to handle these characters effectively.javaString url = "https://www.example.com/my page"; String safeUrl = url.replace(" ", "%20"); System.out.println(safeUrl);
In this snippet, spaces in the URL are replaced by
%20
, converting it to a URL-encoded string:https://www.example.com/my%20page
.
Advanced Replacements Using replaceAll()
Using Regular Expressions
Import the
String
class and initialize a complex string.Employ
replaceAll()
with a regular expression to perform advanced replacements.javaString message = "Contact us at: 000-123-4567 or 000.123.4567"; String normalizedMessage = message.replaceAll("[.-]", ""); System.out.println(normalizedMessage);
This example removes dots and dashes from the contact numbers, making them
0001234567
. Note the use of[.-]
, which is a regular expression character class meaning "any dot or dash."
Conclusion
The replace()
function in Java provides an efficient and straightforward way to manipulate strings by substituting parts of the text with new characters or substrings. Mastering this function enables you to handle everyday coding tasks involving string manipulation more effectively. Whether you're working with simple character replacements or need to tackle more complex patterns with replaceAll()
, Java offers robust solutions for maintaining and processing strings to meet various application needs. As you integrate these skills into your Java programs, appreciate how they contribute to cleaner, more efficient, and error-free code.
No comments yet.