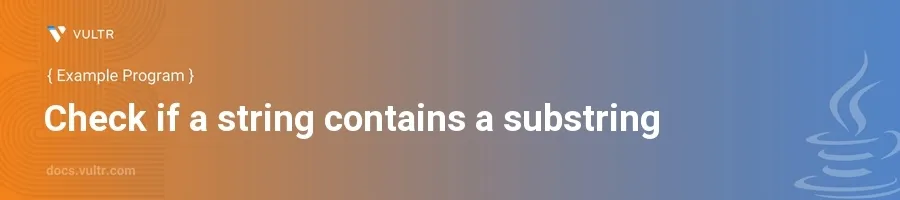
Introduction
In Java programming, string manipulation is a fundamental concept that is frequently utilized in various applications and systems. Checking if a string contains a specific substring is a common operation that can be crucial for tasks such as data validation, parsing, or conditional executions based on text content.
In this article, you will learn how to check if a string contains a substring in Java. You'll explore multiple methods, including the use of standard library functions and handling edge cases effectively. By the end of this piece, you'll be equipped to efficiently implement substring checks in Java using different techniques.
Utilizing the contains()
Method
The simplest and most direct way to check for the presence of a substring within a string in Java is by using the contains()
method available in the String
class. This method returns a boolean value: true
if the sequence of char values is found, or false
otherwise.
Basic Usage of contains()
Define a base string in which you want to search for the substring.
Use the
contains()
method by passing the substring you want to find.javaString baseString = "Hello, welcome to the Java guide!"; boolean result = baseString.contains("Java"); System.out.println("Does the string contain 'Java'? " + result);
This code snippet will output
true
because the substring "Java" is present in the base string.
Consider Case Sensitivity
Consider that the
contains()
method is case-sensitive.To perform a case-insensitive search, convert both strings (the base string and the substring) to the same case (either upper or lower) using
toLowerCase()
ortoUpperCase()
methods before callingcontains()
.javaString baseString = "Hello, welcome to the JAVA guide!"; String subString = "java"; boolean result = baseString.toLowerCase().contains(subString.toLowerCase()); System.out.println("Does the string contain 'java'? " + result);
This code will also return
true
, ensuring the substring check is case-insensitive.
Using indexOf()
Method
The indexOf()
method of the String
class can also determine whether a substring exists within a string. It returns the index of the first occurrence of the specified substring, or -1
if the substring is not found.
Implementing Substring Check with indexOf()
Use
indexOf()
to find the position of the substring in the string.Check if the returned index is greater than
-1
, which indicates the substring is present.javaString baseString = "Searching within strings in Java"; int result = baseString.indexOf("Java"); boolean found = result >= 0; System.out.println("Is 'Java' found within the string? " + found);
This example will print
true
because "Java" is present in the string.
Using Regular Expressions with matches()
For more complex substring detection scenarios, Java's matches()
method can be utilized along with regular expressions. This method checks if the string matches the expression provided.
Using Regular Expressions to Detect Substring
Use the
matches()
method with a regex pattern that includes the substring you are checking for.Notice that
matches()
checks the entire string against the pattern, so include wildcard characters to match any part of the string.javaString baseString = "Java development is versatile"; boolean result = baseString.matches(".*Java.*"); System.out.println("Does the string match 'Java'? " + result);
This outputs
true
because.*
allows for any characters (including none) before and after "Java". This regex effectively checks for the presence of "Java" anywhere in the string.
Conclusion
Checking if a string contains a specific substring is a common task in Java programming, essential for many applications from web development to data processing. By understanding and implementing the methods discussed—contains()
, indexOf()
, and matches()
—you possess powerful tools for string manipulation in Java. Each method offers different advantages depending on the context and specific requirements of your application. Use these techniques to enhance your ability to handle and manipulate strings accurately and efficiently in Java.
No comments yet.