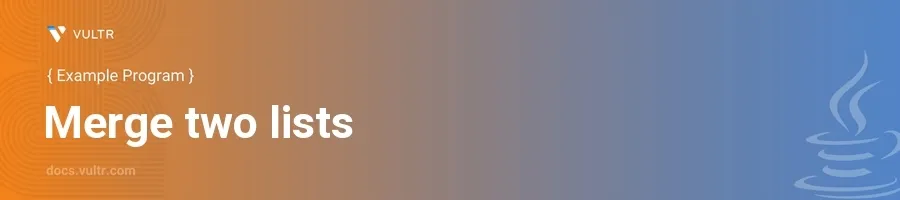
Introduction
Merging two lists in Java is a common operation you might need in various applications, such as combining data from different sources or simply managing lists effectively in a project. This task can be accomplished using different methods depending on the specific requirements of your operation, such as order preservation or the elimination of duplicates.
In this article, you will learn how to merge two lists in Java through several examples. By exploring different methods like using loops, Java 8 streams, and third-party libraries, you grasp the flexibility and power of Java for handling collections. Each example will include code snippets and explanations to ensure you can apply these techniques in your own projects.
Basic Loop Method for Merging Lists
Merging lists using loops is straightforward and does not require any special libraries. This method is perfect when you are looking to understand the basic mechanics of list operations without additional complexity.
Create two sample lists.
Initialize a new list that will store the merged result.
Use a loop to add all elements from both lists to the new list.
javaimport java.util.ArrayList; import java.util.List; public class ListMerger { public static void main(String[] args) { List<String> list1 = new ArrayList<>(); list1.add("Apple"); list1.add("Banana"); List<String> list2 = new ArrayList<>(); list2.add("Orange"); list2.add("Pineapple"); List<String> mergedList = new ArrayList<>(list1); mergedList.addAll(list2); System.out.println("Merged List: " + mergedList); } }
This code initially creates two lists,
list1
andlist2
, and then merges them intomergedList
. TheaddAll()
method from theArrayList
class is used to add all elements fromlist2
tomergedList
which already contains the elements fromlist1
.
Java 8 Stream API for Merging Lists
The Stream API, introduced in Java 8, offers a more functional approach to handle collections. It is particularly useful for merging lists as it simplifies the code and improves readability.
Use the
Stream
API to concatenate lists.Collect the results back into a new list.
javaimport java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; import java.util.stream.Stream; public class ListMerger { public static void main(String[] args) { List<String> list1 = new ArrayList<>(); list1.add("Apple"); list1.add("Banana"); List<String> list2 = new ArrayList<>(); list2.add("Orange"); list2.add("Pineapple"); List<String> mergedList = Stream.concat(list1.stream(), list2.stream()) .collect(Collectors.toList()); System.out.println("Merged List using Streams: " + mergedList); } }
In this snippet,
Stream.concat()
is used to create a continuous stream by concatenating the streams oflist1
andlist2
. Thecollect()
method withCollectors.toList()
then transforms this stream back into a list.
Using Third-Party Libraries
Sometimes you are not restricted to using only JDK and can utilize third-party libraries that provide more functionality. Apache Commons Collections and Google Guava are popular among Java developers for advanced collection handling.
Use
ListUtils.union()
from Apache Commons Collections to merge lists.Use
Iterables.concat()
from Google Guava for the same purpose.javaimport org.apache.commons.collections4.ListUtils; import com.google.common.collect.Iterables; import java.util.Arrays; import java.util.List; public class ListMerger { public static void main(String[] args) { List<String> list1 = Arrays.asList("Apple", "Banana"); List<String> list2 = Arrays.asList("Orange", "Pineapple"); List<String> mergedListCommons = ListUtils.union(list1, list2); Iterable<String> mergedListGuava = Iterables.concat(list1, list2); System.out.println("Merged List using Apache Commons: " + mergedListCommons); System.out.println("Merged List using Guava: " + mergedListGuava); } }
ListUtils.union()
creates a new list containing the union of the two lists whileIterables.concat()
provides a view that concatenates two iterables together.
Conclusion
Merging two lists in Java can be done in various ways, each suitable for different scenarios depending on your project requirements. From using simple loops and the Stream API for direct manipulation, to employing powerful third-party libraries that provide additional functionality, Java offers numerous options for efficiently handling list operations. Utilizing these different methods allows for flexible code architectures and can improve both performance and readability in your Java applications. Tailor your approach to the needs of your project and harness the power of Java to manage collections effectively.
No comments yet.