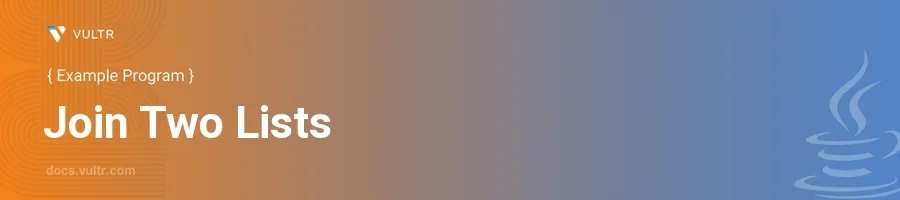
Introduction
Java provides several ways to join two lists, a common operation in programming when handling collections of data. This operation is crucial in scenarios where data from different sources needs to be consolidated or when multiple results need to be aggregated.
In this article, you will learn how to join two lists in Java using different methods. Explore practical examples using plain loops, collection utilities, and Java 8 Stream API, each tailored to integrate lists effectively depending on the situation and your specific needs.
Joining Lists Using Loops
Plain For Loop Method
Create two lists that will be joined.
Initialize a new list to store the combined results.
Use a for loop to add elements from the first and then the second list.
javaList<String> listOne = Arrays.asList("Java", "Python"); List<String> listTwo = Arrays.asList("C#", "JavaScript"); List<String> combinedList = new ArrayList<>(); for (String element : listOne) { combinedList.add(element); } for (String element : listTwo) { combinedList.add(element); } System.out.println("Combined List: " + combinedList);
This code first adds all elements from
listOne
tocombinedList
, followed by all elements fromlistTwo
. As a result,combinedList
contains all elements from both lists in order.
Using the addAll() Method
Declare and initialize two lists.
Create a new list to store the concatenated results.
Utilize the
addAll()
method to join the lists.javaList<String> listOne = Arrays.asList("Apple", "Orange"); List<String> listTwo = Arrays.asList("Banana", "Grape"); List<String> combinedList = new ArrayList<>(listOne); combinedList.addAll(listTwo); System.out.println("Combined List: " + combinedList);
In this example,
combinedList
initially copies all elements fromlistOne
and subsequently appends all elements fromlistTwo
usingaddAll()
.
Joining Lists Using Java 8 Stream API
Stream.concat() Method
Ensure you have two lists to combine.
Use
Stream.concat()
from Java 8 to merge the streams of both lists, then collect results into a new list.javaList<String> listOne = Arrays.asList("Blue", "Green"); List<String> listTwo = Arrays.asList("Yellow", "Red"); List<String> combinedList = Stream.concat(listOne.stream(), listTwo.stream()) .collect(Collectors.toList()); System.out.println("Combined List via Stream: " + combinedList);
Stream.concat()
merges two streams created fromlistOne
andlistTwo
. Usingcollect()
, these are gathered into a combined list.
Using flatMap() for More Complex Structures
Assume the presence of two lists that potentially contain lists as elements.
Utilize
flatMap()
to handle and flatten nested list structures into a single list.javaList<String> innerList1 = Arrays.asList("Spring", "Summer"); List<String> innerList2 = Arrays.asList("Fall", "Winter"); List<List<String>> outerList1 = new ArrayList<>(); outerList1.add(innerList1); List<List<String>> outerList2 = new ArrayList<>(); outerList2.add(innerList2); List<String> combinedList = Stream.of(outerList1, outerList2) .flatMap(Collection::stream) .flatMap(Collection::stream) .collect(Collectors.toList()); System.out.println("Combined List from Nested Lists: " + combinedList);
Here,
flatMap()
is essential to flatten fromList<List<String>>
toList<String>
, combining all individual season names into a single list.
Conclusion
Combining two lists in Java can be achieved through straightforward loops, utility methods like addAll()
, or using the powerful Stream API for more complex and flexible operations. Each approach has its use cases depending on the complexity and the context in which data needs to be merged. Practice these techniques to enhance your collection handling skills and ensure your Java programs can efficiently process grouped data. Remember, the right method helps maintain clarity and performance of your code.
No comments yet.