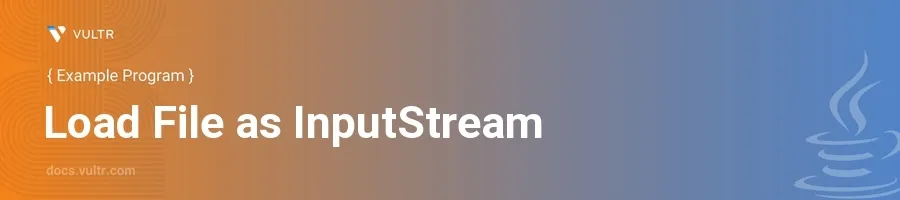
Introduction
Java provides several methods to handle files, and one of the most versatile ways to work with files is through the use of InputStream. An InputStream in Java is an abstract class that serves as the superclass for all classes representing an input stream of bytes. This approach is particularly useful when you need to read a file as an InputStream byte by byte, which is common in network communications, file processing applications, and when converting a file to an InputStream for further manipulation.
In this article, you will learn how to load and read files as InputStreams in Java. Explore different methods, including using the FileInputStream
class and loading files from the classpath, to handle file loading efficiently in multiple real-world programming scenarios.
Loading a File with FileInputStream
Read a File Using FileInputStream into a Byte Array
Create a
FileInputStream
instance pointing to the desired file.Determine the file size and read its contents into a byte array.
javaFileInputStream fileInputStream = new FileInputStream("example.txt"); byte[] data = new byte[fileInputStream.available()]; int bytesRead = fileInputStream.read(data); fileInputStream.close();
This code snippet initializes a
FileInputStream
for the file namedexample.txt
. It creates a byte array sized to the number of available bytes in the stream (typically the file size) and reads the file content into this byte array. The stream is then closed to free resources. This method is ideal when you need to convert a file to an InputStream and then process it further in your Java application.
Handling Larger Files
Use a loop to read chunks of the file at a time.
Employ a buffer to hold data segments.
javaFileInputStream inputStream = new FileInputStream("largeFile.txt"); byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = inputStream.read(buffer)) != -1) { // Process the buffer } inputStream.close();
For larger files, reading the entire file into memory might not be feasible. This example demonstrates how to read 1024 bytes at a time using a buffer array. The
read
function fills the buffer and returns the number of bytes actually read, and the loop continues until the end of the file is reached.
Reading Files from Classpath
Using getResourceAsStream()
Use
getClass().getResourceAsStream()
to read files from the classpath.This is particularly useful in applications packaged into JAR files.
javaInputStream resourceStream = getClass().getResourceAsStream("/config.properties"); Properties properties = new Properties(); properties.load(resourceStream); resourceStream.close();
The method
getResourceAsStream()
loads files that are accessible via the classpath, making it ideal for accessing resources in JAR files. This example shows how to load a properties file. Handling streams always requires closing them to free up system resources.
Advanced InputStream Handling
Reading with BufferedInputStream
Enhance performance using
BufferedInputStream
.Wrap a
FileInputStream
to read the file more efficiently.javaFileInputStream fileIn = new FileInputStream("example.txt"); BufferedInputStream bufferedIn = new BufferedInputStream(fileIn); int singleByte = bufferedIn.read(); while (singleByte != -1) { // Process the single byte singleByte = bufferedIn.read(); } bufferedIn.close(); fileIn.close();
BufferedInputStream
wraps another input stream and provides buffering capabilities to minimize the number of real reads from the underlying disk. It’s beneficial for improving performance when reading files byte by byte.
Converting Between InputStream and File in Java
Converting a File to an InputStream
Use
FileInputStream
to create anInputStream
from a file.This is useful when you need to process a file as a stream, such as for network transfers or memory-efficient file handling.
javaFile file = new File("example.txt"); InputStream inputStream = new FileInputStream(file);
This example initializes a
FileInputStream
to read the fileexample.txt
as anInputStream
. It allows efficient byte-by-byte or buffered reading.
Converting an InputStream to a File
Use
FileOutputStream
to write the contents of anInputStream
to a file.A buffer ensures efficient data transfer.
javaInputStream inputStream = new FileInputStream("example.txt"); FileOutputStream outputStream = new FileOutputStream("output.txt"); byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } inputStream.close(); outputStream.close();
This method reads data from an
InputStream
in chunks and writes it to a new file. Closing both streams ensures resource management.
Conclusion
Loading and reading files in Java using InputStreams offers a flexible approach suitable for a variety of file-handling scenarios. By mastering the use of FileInputStream
, BufferedInputStream
, and accessing classpath resources with getResourceAsStream()
, you ensure robust file manipulation capabilities in your Java applications. Implementing these techniques helps manage file input efficiently, catering to small and large files and maintaining performance integrity in Java-based software projects.
Whether you are reading a file as an InputStream, converting a file to an InputStream, or handling FileInputStream from the classpath, these methods ensure seamless file operations in Java.
No comments yet.