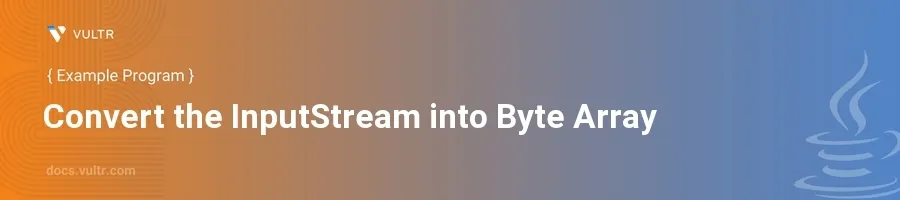
Introduction
The conversion of an InputStream into a byte array in Java is a common requirement when working with I/O operations. This task becomes pertinent when handling data fetched from files, network resources, or any other input source that supports streaming. Typically, converting this stream into a byte array makes it easier to manipulate, store, or transmit the data as needed.
In this article, you will learn how to efficiently convert an InputStream into a byte array in Java through different examples. Explore various methods, including using Apache Commons IO libraries, and delve into some java-native approaches to achieve this. These illustrations serve practical applications in real-world programming scenarios, enabling you to handle data streams effectively.
Java Native Approach
Using ByteArrayOutputStream
Read from the InputStream.
Write into a ByteArrayOutputStream.
Convert the ByteArrayOutputStream to a byte array.
javaimport java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; public class InputStreamConverter { public static byte[] toByteArray(InputStream input) throws IOException { ByteArrayOutputStream output = new ByteArrayOutputStream(); byte[] buffer = new byte[1024]; int length; while ((length = input.read(buffer)) != -1) { output.write(buffer, 0, length); } return output.toByteArray(); } }
This method reads bytes from an InputStream into a buffer, then writes them into a ByteArrayOutputStream. By repeatedly reading and writing, it captures all data from the InputStream. Finally, it converts the ByteArrayOutputStream into a byte array.
Utilizing Java 9 TransferTo Method
Use the
transferTo
method from an InputStream directly into a ByteArrayOutputStream.Convert the ByteArrayOutputStream's content to a byte array.
javaimport java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; public class StreamConverterJava9 { public static byte[] toByteArray(InputStream input) throws IOException { ByteArrayOutputStream output = new ByteArrayOutputStream(); input.transferTo(output); return output.toByteArray(); } }
The
transferTo
method introduced in Java 9 simplifies streaming from an InputStream to an OutputStream. The method handles buffering internally, reducing the amount of boilerplate code.
Using Third-Party Libraries
Utilizing Apache Commons IO Library
Include the Apache Commons IO library in your project.
Use the
IOUtils.toByteArray(InputStream input)
method.javaimport java.io.IOException; import java.io.InputStream; import org.apache.commons.io.IOUtils; public class InputStreamWithApache { public static byte[] toByteArray(InputStream input) throws IOException { return IOUtils.toByteArray(input); } }
Apache Commons IO simplifies many operations with streams. The
toByteArray
method is a high-level utility that reads all content from an InputStream and returns it as a byte array. Ensure you add the Commons IO dependency to your project:xml<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-io</artifactId> <version>2.8.0</version> </dependency>
This code snippet shows how to add Apache Commons IO to a Maven project using the
pom.xml
file.
Conclusion
The conversion of InputStream to byte array is a necessary skill when dealing with I/O operations in Java. The methods described emphasize native Java solutions and an external library approach with Apache Commons IO. Each method has its own best-case usage scenarios—the Java native approach provides fine control and doesn't rely on external dependencies, while the Apache Commons IO library offers a more concise and readable solution. Depending on your specific requirements and project environment, choose the method that best aligns with your development practices. By mastering these techniques, you enhance your ability to handle data efficiently in your Java applications.
No comments yet.