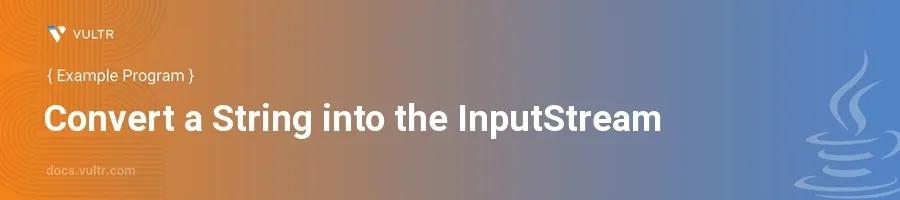
Introduction
Converting a string to an InputStream
in Java is a relevant operation when working with I/O operations, APIs, or any situation where data needs to be processed in the form of streams. This capability is especially useful when your Java code interacts with libraries that require input to be in the form of streams rather than raw strings.
In this article, you will learn how to convert a string into an InputStream
using different methods in Java. You'll explore examples that utilize the java.io
package to effectively transform string data into a stream and see how these streams integrate with other Java functionalities for robust app development.
Converting with ByteArrayInputStream
Basic Conversion using ByteArrayInputStream
Import the necessary classes.
Create an instance of
ByteArrayInputStream
using the byte array of the string.javaimport java.io.ByteArrayInputStream; import java.io.InputStream; import java.nio.charset.StandardCharsets; String str = "Example String"; InputStream stream = new ByteArrayInputStream(str.getBytes(StandardCharsets.UTF_8));
In this code snippet, you convert the string
str
into a byte array with UTF-8 encoding and then instantiate aByteArrayInputStream
with this byte array. The resultingInputStream
stream
can now be used as a regular input stream in Java.
Using ByteArrayInputStream in Functions
Incorporate the conversion into a reusable function.
Demonstrate using this function in stream operations.
javaimport java.io.ByteArrayInputStream; import java.io.InputStream; import java.nio.charset.StandardCharsets; public InputStream stringToInputStream(String input) { return new ByteArrayInputStream(input.getBytes(StandardCharsets.UTF_8)); } public static void main(String[] args) { String myString = "Hello, World!"; InputStream myStream = stringToInputStream(myString); int data = myStream.read(); while (data != -1) { System.out.print((char) data); data = myStream.read(); } myStream.close(); }
This example defines a method,
stringToInputStream
, which performs the conversion. In themain
method, the string "Hello, World!" is converted to anInputStream
, and we read from this stream byte by byte until the end, printing each character. This mimics how you might process stream data in real applications.
Converting with Apache Commons IO
Utilizing IOUtils.toInputStream
Add Apache Commons IO library to your project.
Use the
IOUtils.toInputStream()
method to convert the string.If you’re working on a Maven project, include the following in your
pom.xml
:xml<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-io</artifactId> <version>2.8.0</version> </dependency>
Now use the library to convert a string into an
InputStream
:javaimport org.apache.commons.io.IOUtils; import java.io.InputStream; import java.nio.charset.StandardCharsets; String str = "Another Example"; InputStream stream = IOUtils.toInputStream(str, StandardCharsets.UTF_8);
This snippet converts the string
str
into anInputStream
using theIOUtils.toInputStream()
method with UTF-8 encoding specified. This can be a more straightforward approach than manually handling byte arrays, especially beneficial in larger projects or where Apache Commons IO is already a dependency.
Conclusion
Converting a string to an InputStream
in Java is a straightforward task that can be achieved through various methods, including using the standard Java libraries like java.io.ByteArrayInputStream
or external libraries such as Apache Commons IO. Understanding how to perform this conversion effectively allows for greater flexibility in your application, letting you interface smoothly with APIs and libraries that process data in stream formats. By integrating these conversion strategies into your Java projects, you uphold strong data handling practices which contribute to building robust and efficient applications.
No comments yet.