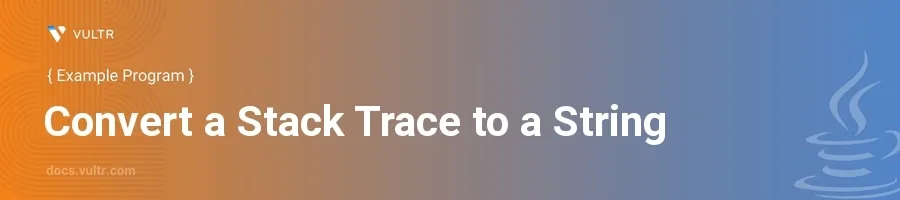
Introduction
When debugging Java applications, capturing a stack trace is a critical step for diagnosing issues. Often, it’s necessary to convert the stack trace into a string format to easily log it or communicate the error details through different platforms like logging frameworks, emails, or databases. This ability lets developers analyze exceptions retrospectively which is essential during the post-mortem of complex issues.
In this article, you will learn how to convert a stack trace to a string using Java. Understand different methodologies and examples that illustrate capturing, manipulating, and converting stack trace data from exceptions. Discover how to effectively utilize built-in Java classes and third-party libraries to streamline error handling in your Java applications.
Methods to Convert Stack Trace to a String
Using StringWriter and PrintWriter
Make use of Java's
java.io.StringWriter
andjava.io.PrintWriter
classes.Catch an exception and process it to extract its stack trace.
javaimport java.io.PrintWriter; import java.io.StringWriter; public class StackTraceToString { public static void main(String[] args) { try { int result = 10 / 0; // This will cause an ArithmeticException } catch (Exception e) { StringWriter sw = new StringWriter(); PrintWriter pw = new PrintWriter(sw); e.printStackTrace(pw); String stackTraceAsString = sw.toString(); System.out.println("Stack Trace as String:\n" + stackTraceAsString); } } }
This code snippet catches an
ArithmeticException
and usesStringWriter
andPrintWriter
to write the exception's stack trace into a string. The process involves creating aPrintWriter
object withStringWriter
as an argument, which gathers the stack trace into a string buffer that can be converted into a string.
Using Exception's StackTraceElement Array
Exploit the
getStackTrace()
method of theThrowable
class.Build a string from the stack trace elements.
javapublic class StackTraceElementsToString { public static void main(String[] args) { try { int result = 10 / 0; // This will cause an ArithmeticException } catch (Exception e) { StackTraceElement[] elements = e.getStackTrace(); StringBuilder sb = new StringBuilder(); for (StackTraceElement element : elements) { sb.append(element.toString()); sb.append("\n"); } String stackTraceAsString = sb.toString(); System.out.println("Stack Trace as String:\n" + stackTraceAsString); } } }
In this example, the stack trace of the captured exception is retrieved as an array of
StackTraceElement
. Each element is converted to string format and appended to aStringBuilder
. This approach gives you more control over the formatting of the stack trace string.
Using Apache Commons Lang
Utilize the
ExceptionUtils.getStackTrace(Throwable)
method from the Apache Commons Lang library.Add the Apache Commons Lang dependency to your project.
javaimport org.apache.commons.lang3.exception.ExceptionUtils; public class StackTraceUsingApacheCommons { public static void main(String[] args) { try { int result = 10 / 0; // This will cause an ArithmeticException } catch (Exception e) { String stackTraceAsString = ExceptionUtils.getStackTrace(e); System.out.println("Stack Trace as String:\n" + stackTraceAsString); } } }
To use this method, make sure to include the Apache Commons Lang in your project. This library simplifies the process by providing a one-line method call that handles the conversion of an exception's stack trace to a string. This is helpful for maintaining cleaner code and focusing on higher-level application logic.
Conclusion
Converting a stack trace to a string in Java assists in logging and error reporting, which are crucial for debugging and maintaining applications. By implementing the techniques discussed, you ensure that error diagnostics are not only preserved but are also readable and manageable. Whether you choose native Java approaches with StringWriter
and PrintWriter
or utilize powerful libraries like Apache Commons Lang, you improve the effectiveness of your error handling processes. These skills foster better monitoring, faster debugging, and ultimately more robust Java applications.
No comments yet.