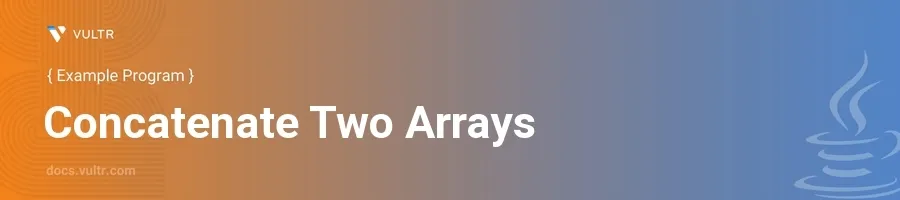
Introduction
Java, known for its robust handling of objects and classes, offers various ways to manage and manipulate arrays. One common task in Java programming is concatenating two arrays - combining them into a single array. This operation is useful in numerous situations, from data processing to everyday programming tasks where you need to merge data stored in separate arrays.
In this article, you will learn how to concatenate two arrays in Java using different methods. Explore straightforward loops and advanced methods involving system functions to achieve your goal. By the end of this guide, you will be able to implement these techniques in your own Java projects, reinforcing your understanding of array handling and manipulation.
Basic Concatenation Using Loops
Concatenating two arrays can be approached in the simplest form by using loops to manually iterate through each array and add their elements to a new, larger array. This method gives you a high level of control over the process and is easy to understand for beginners.
Example: Concatenating Integer Arrays
Define two arrays of integers that you wish to concatenate.
Create a new array that has the combined length of both arrays.
Use a loop to copy elements from the first array into the new array.
Use another loop to add elements from the second array to the new array, starting from where the first left off.
javaint[] array1 = {1, 2, 3}; int[] array2 = {4, 5, 6}; int[] concatenatedArray = new int[array1.length + array2.length]; int i = 0; for (; i < array1.length; i++) { concatenatedArray[i] = array1[i]; } for (int j = 0; j < array2.length; i++, j++) { concatenatedArray[i] = array2[j]; }
This code first iterates over
array1
, assigning its elements to the newconcatenatedArray
. After finishing witharray1
, it continues witharray2
, starting from where it left off. This ensures that all elements from both arrays are included inconcatenatedArray
.
Advanced Concatenation Using System Functions
For a more efficient approach when performance or readability is a concern, you can use Java's built-in system functions. The System.arraycopy()
method is particularly useful for concatenating arrays because it is designed to efficiently copy arrays.
Example: Concatenating String Arrays
Define two string arrays.
Initialize a new string array that can hold all elements from both arrays.
Use
System.arraycopy()
to copy the first array into the new array.Use
System.arraycopy()
again to copy the second array into the new array, starting right after the last element of the first array.javaString[] array1 = {"Hello", "World"}; String[] array2 = {"Concatenation", "Example"}; String[] concatenatedArray = new String[array1.length + array2.length]; System.arraycopy(array1, 0, concatenatedArray, 0, array1.length); System.arraycopy(array2, 0, concatenatedArray, array1.length, array2.length);
Here,
System.arraycopy()
first copies all elements fromarray1
intoconcatenatedArray
. Then it continues to copy elements fromarray2
, starting at the index immediately following the last index filled byarray1
. This method is faster than a loop, especially for larger arrays, and keeps the code concise.
Conclusion
Concatenating arrays in Java is a fundamental skill that enhances flexibility and efficiency in data handling tasks. Whether using basic loops for greater control or advanced system functions for better performance and readability, mastering array concatenation allows you to seamlessly integrate separate data sets in your applications. Implement these methods in your array manipulation toolkit to ensure your applications are robust and efficient.
No comments yet.