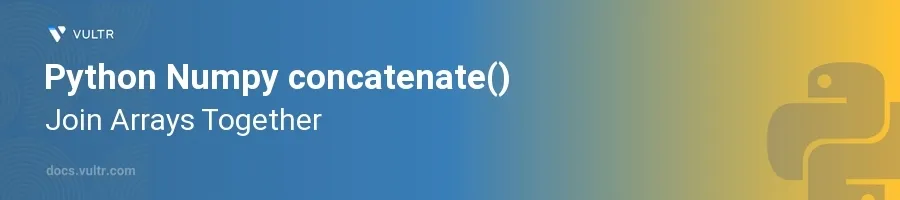
Introduction
The concatenate()
function in the Numpy library is pivotal for combining arrays in Python. It serves a fundamental role in scientific and numerical computing by allowing the merging of arrays along a specified axis, directly affecting data structure and preparation. Understanding how to efficiently join arrays using concatenate()
can substantially optimize your data manipulation tasks in Python.
In this article, you will learn how to effectively use the concatenate()
function to join arrays together. Explore the versatility of this function in different scenarios such as concatenating two or more arrays along various axes, handling arrays of different dimensions, and implementing this within practical examples.
Basics of Numpy concatenate()
Joining Two Arrays Along Default Axis
Import the Numpy library.
Define two or more arrays to concatenate.
Apply the
concatenate()
function.pythonimport numpy as np array1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) result = np.concatenate((array1, array2)) print(result)
This code concatenates
array1
andarray2
along the default axis (axis 0 for 1D arrays), producing a single merged array.
Concatenating Along a Specific Axis
Ensure the arrays have the same shape or are compatible along the specified axis.
Use the
axis
parameter inconcatenate()
to define the axis of concatenation.pythonarray1 = np.array([[1, 2], [3, 4]]) array2 = np.array([[5, 6], [7, 8]]) result = np.concatenate((array1, array2), axis=1) print(result)
Here,
array1
andarray2
are two-dimensional, and by settingaxis=1
, the arrays are concatenated side by side (column-wise).
Handling Arrays with Different Dimensions
Using np.newaxis
to Adjust Dimensions
Use
np.newaxis
to increase the dimensionality of the array.Concatenate the adjusted arrays as needed.
pythonarray1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) array1_new = array1[:, np.newaxis] result = np.concatenate((array1_new, array2[:, np.newaxis]), axis=1) print(result)
This snippet reshapes both
array1
andarray2
into column vectors before concatenating them horizontally.array1[:, np.newaxis]
changesarray1
from 1D to a 2D array (specifically, a column vector).
Advanced Concatenation Techniques
Concatenating More Than Two Arrays
Collect all arrays to concatenate into a tuple or list.
Pass the collection of arrays to
concatenate()
.pythonarray1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) array3 = np.array([7, 8, 9]) result = np.concatenate((array1, array2, array3), axis=0) print(result)
The example concatenates
array1
,array2
, andarray3
along the default axis, creating a new array that combines the elements of all three arrays.
Conclusion
The concatenate()
function in Numpy is a robust tool for array manipulation, allowing arrays to be joined efficiently along different dimensions. By mastering this function, you enhance your ability to handle complex data transformations, making your data analysis tasks more streamlined and efficient. Remember to always consider the dimensions and the axes along which you're concatenating to ensure the arrays align properly. With this knowledge, you can now manipulate arrays expertly in your projects, ensuring data integrity and optimal processing.
No comments yet.