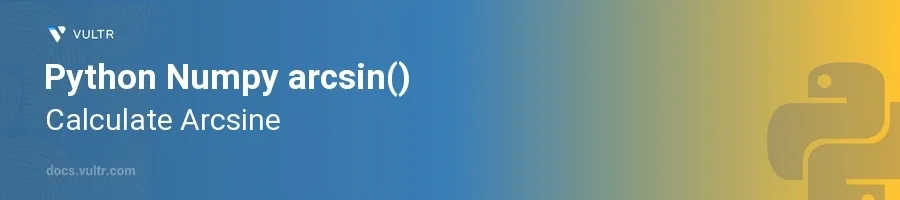
Introduction
The Python Numpy library provides a comprehensive mathematical function called arcsin()
, which calculates the arcsine of an input array, element-wise. This function returns the inverse sine values for each element, allowing for complex mathematical computations that are crucial in fields such as physics and engineering.
In this article, you will learn how to effectively leverage the arcsin()
function from the Numpy library to calculate the arcsine of various types of data. Explore practical examples that demonstrate how to manipulate arrays of angles and process these values to achieve accurate mathematical results.
Working with arcsin() Function
Basics of arcsin() Function
Import the numpy library.
Create an example array containing sine values.
Apply the
np.arcsin()
function.pythonimport numpy as np sine_values = np.array([-1, -0.5, 0, 0.5, 1]) angles = np.rad2deg(np.arcsin(sine_values)) print(angles)
This code calculates the arcsine of each sine value in the array
sine_values
. The result fromnp.arcsin()
is in radians, which is then converted to degrees usingnp.rad2deg()
for better interpretability. The output will be an array of angles in degrees corresponding to the input sine values.
Dealing with Arcsine Domain
Understand that
np.arcsin()
requires input values in the range [-1, 1].Handle inputs outside this domain to prevent runtime errors.
pythonimport numpy as np invalid_values = np.array([-2, -1.5, 2]) valid_mask = (invalid_values >= -1) & (invalid_values <= 1) correct_results = np.arcsin(invalid_values[valid_mask]) print(correct_results)
Here,
valid_mask
is used to filter the arrayinvalid_values
so that only the values within the domain of arcsine are processed bynp.arcsin()
. This technique prevents the function from throwing a domain error.
Practical Application: Calculating Angles
Use
arcsin()
function in practical physics problem-solving.Define the problem context and input data.
Calculate the output using the
arcsin()
function.pythonimport numpy as np # Incidence angles for refracted light, sine values given refraction_indices = np.array([0.87, 0.65]) incidence_angles = np.rad2deg(np.arcsin(refraction_indices)) print(incidence_angles)
This script calculates the incidence angles of light given the refraction index using Snell's law. The arcsine of the refracted index values gives the incidence angles in radians, which are then converted to degrees.
Conclusion
The arcsin()
function in Numpy is indispensable for computing inverse sine in scientific and engineering disciplines. By understanding how to handle inputs appropriately, especially considering its limited domain, you can effectively use this function to analyze and interpret physical phenomena and mathematical problems. The examples provided demonstrate its utility in real-world applications, ensuring that your computational solutions are both robust and reliable. Whether you are looking to perform advanced mathematical computations or solve geometry-related problems, the arcsin()
function is a reliable tool to achieve precise results.
No comments yet.