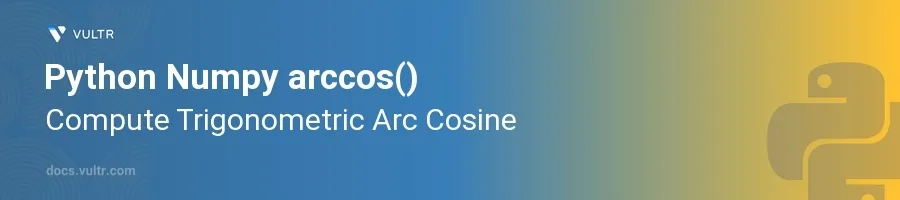
Introduction
The numpy.arccos()
function is an essential part of the NumPy library in Python, allowing users to compute the trigonometric arc cosine, or inverse cosine, of all the elements in an input array. This function is widely used in fields that require geometric or trigonometric calculations, such as physics, engineering, and computer graphics.
In this article, you will learn how to use the numpy.arccos()
function effectively. Delve into scenarios showcasing practical applications of arc cosine calculations on single values, arrays, and within real-world contexts. Discover how to interpret the results given by numpy.arccos()
and handle different types of data effectively.
Understanding numpy.arccos()
Single Value Calculation
Import the NumPy library.
Choose a numeric value between -1 and 1 for cosine input.
Apply
numpy.arccos()
to compute the arc cosine.pythonimport numpy as np cos_value = 0.5 radian_result = np.arccos(cos_value) print("Arc Cosine (radians):", radian_result) degree_result = np.degrees(radian_result) print("Arc Cosine (degrees):", degree_result)
The above code calculates the arc cosine of 0.5, returning the result in both radians and degrees. The function
np.degrees()
is used to convert the result from radians to degrees.
Application on Arrays
Define an array with cosine values within the valid range from -1 to 1.
Use
numpy.arccos()
to compute arc cosines for the entire array.pythonimport numpy as np array_cos_values = np.array([-1, 0, 0.5, 1]) array_radian_results = np.arccos(array_cos_values) print("Arc Cosine (radians):", array_radian_results)
This snippet evaluates the arc cosine of each element in the array
array_cos_values
. Results for multiple values are processed efficiently in a single operation.
Handling Special Cases
Be aware of domain errors that occur when input values are out of the [-1, 1] range.
Handle these cases to prevent runtime errors using conditional checks.
pythonimport numpy as np def safe_arccos(x): if -1 <= x <= 1: return np.arccos(x) else: return None test_values = [-2, -1, 0, 1, 2] results = [safe_arccos(x) for x in test_values] print("Results:", results)
This protective function,
safe_arccos()
, checks if the input is within the allowable range before computing its arc cosine. Outputs are either a valid result orNone
for out-of-range values.
Conclusion
The numpy.arccos()
function is a robust tool for computing the arc cosine values of numbers, facilitating work with trigonometric functions in numerical computing. By understanding and implementing the techniques discussed, you enhance your ability to achieve accurate results in scientific calculations. Whether working with single values, arrays, or incorporating conditional logic to handle special cases, numpy.arccos()
proves itself as a crucial component in Python's numerical toolkit.
No comments yet.