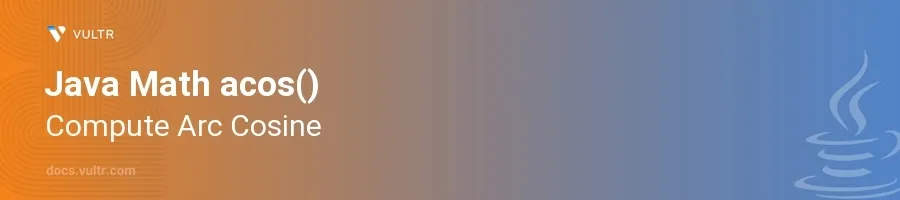
Introduction
The Math.acos()
method in Java is used to compute the arc cosine of a given angle, returned in radians. This method is helpful in various mathematical calculations where you need to derive the angle from its cosine value, primarily in fields of geometry, physics, and engineering simulations.
In this article, you will learn how to utilize the Math.acos()
method in Java effectively. Explore practical examples to understand how to apply this method in real-world programming challenges, and get insights into handling edge cases and interpreting the results accurately.
Basic Usage of acos()
Compute Arc Cosine of a Value
Recognize that the input value must lie in the range [-1, 1].
Call the
Math.acos()
method with a valid input and observe the output.javadouble result = Math.acos(0.5); System.out.println("Arc cosine of 0.5 is: " + result + " radians");
This code calculates the arc cosine of 0.5, which should return a value in radians. The result approximates to 1.047 radians, which is equivalent to 60 degrees.
Range of Input and Output
Understand that inputs outside the range [-1, 1] will result in NaN (Not a Number).
Experiment with values to see the effects.
javadouble invalidResult = Math.acos(1.5); System.out.println("Arc cosine of 1.5 is: " + invalidResult);
In this snippet, the input 1.5 is outside the valid range, so
Math.acos()
returns NaN, indicating an undefined or unrepresentable result.
Using acos() in Geometric Calculations
Calculate the Angle Between Two Vectors
Define two vectors in the plane.
Use the dot product and magnitude of vectors to find the cosine of the angle.
Apply
Math.acos()
to calculate the angle in radians.javadouble[] vectorA = {3, 4}; double[] vectorB = {4, 3}; double dotProduct = vectorA[0] * vectorB[0] + vectorA[1] * vectorB[1]; double magnitudeA = Math.sqrt(vectorA[0]*vectorA[0] + vectorA[1]*vectorA[1]); double magnitudeB = Math.sqrt(vectorB[0]*vectorB[0] + vectorB[1]*vectorB[1]); double angle = Math.acos(dotProduct / (magnitudeA * magnitudeB)); System.out.println("Angle between vectors is: " + angle + " radians");
This example calculates the angle between two vectors using the cosine rule. The result is the angle in radians.
Conclusion
The Math.acos()
function in Java is a critical tool for computing the arc cosine of a value, which helps derive angles from the cosine in mathematical and geometric computations. Understand its input constraints to avoid common mistakes like NaN results. Employ this method in diverse situations, ranging from simple angle calculations to more complex applications like finding the angle between vectors. By mastering Math.acos()
, enhance your mathematical problem-solving skills in Java programming.
No comments yet.