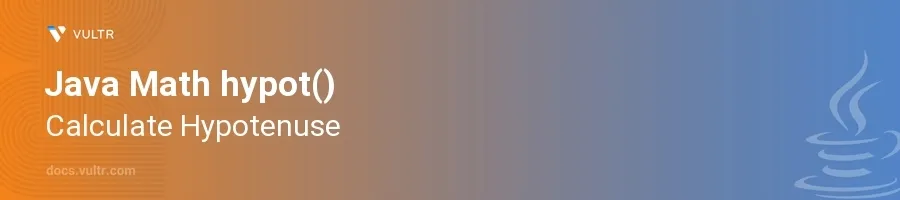
Introduction
The Math.hypot()
method in Java is a robust and precise function for calculating the hypotenuse of a right-angled triangle, using the lengths of its two other sides. By leveraging the Pythagorean theorem under the hood, this method ensures accuracy, especially in dealing with large and diverse data types, where direct computation could lead to errors due to floating-point arithmetic issues.
In this article, you will learn how to utilize the Math.hypot()
method to calculate the hypotenuse in various scenarios. Discover not only basic operations but also practical uses in real-world applications involving triangle calculations and distance measurements.
Basics of Math.hypot()
Calculate a Simple Hypotenuse
Define two sides of a right-angled triangle.
Use the
Math.hypot()
function.javadouble side1 = 3.0; double side2 = 4.0; double hypotenuse = Math.hypot(side1, side2); System.out.println("Hypotenuse: " + hypotenuse);
This code calculates the hypotenuse of a triangle with sides of lengths 3.0 and 4.0. Since the sides adhere to the Pythagorean triplet (3, 4, 5),
Math.hypot()
returns 5.0.
Handling Different Data Types
Note
Math.hypot()
accepts anydouble
type values, offering flexibility.Declare variables with real numbers.
javadouble sideX = 8.0; double sideY = 6.0; double hypotenuseResult = Math.hypot(sideX, sideY); System.out.println("Hypotenuse: " + hypotenuseResult);
Here, whether sides represent integers, floating points, or double-precision numbers,
Math.hypot()
computes the hypotenuse correctly.
Practical Applications of Math.hypot()
Measuring Distance in a Coordinate Plane
Understand that the distances between two points (x1, y1) and (x2, y2) can be viewed as a hypotenuse calculation.
Calculate the Euclidean distance between points.
javadouble x1 = 1.0, y1 = 1.0; double x2 = 4.0, y2 = 5.0; double distance = Math.hypot(x2 - x1, y2 - y1); System.out.println("Distance: " + distance);
The computation
Math.hypot(x2 - x1, y2 - y1)
effectively measures the distance between two points in a 2D space.
Application in Game Development
Acknowledge that in gaming physics, determining the distance between objects is vital for collision detection.
Implement distance calculation using
Math.hypot()
.javadouble object1_X = 10.0, object1_Y = 20.0; double object2_X = 15.0, object2_Y = 25.0; double objectDistance = Math.hypot(object2_X - object1_X, object2_Y - object1_Y); System.out.println("Object Distance: " + objectDistance);
This example computes the distance between two objects in a game's environment, which could help in programming mechanics like triggering events when objects are within a certain proximity.
Conclusion
Utilize the Math.hypot()
method in Java for precise and accurate hypotenuse calculation and distance measurement. Its reliability in handling potential pitfalls of floating-point arithmetic makes it indispensable in scientific calculations, game development, and computational geometry among other fields. By integrating the methods discussed, elevation of code effectiveness and operational accuracy in mathematical computations is ensured.
No comments yet.